How to Assign Default Value to a Variable Using Bash
Last updated: March 18, 2024
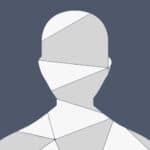
It's finally here:
>> The Road to Membership and Baeldung Pro .
Going into ads, no-ads reading , and bit about how Baeldung works if you're curious :)
We’ve started a new DevOps area on the site. If you’re interested in writing about DevOps, check out the Contribution Guidelines .

1. Overview
When dealing with variables in shell scripting , it’s important to account for the fact that a variable may have one of several states:
- assigned value
- null string
Thus, to avoid unexpected errors, we can use the ${param:-param} expression along with similar expressions to assign a default value to a variable under specific conditions.
In this tutorial, we’ll explore how to assign a default value to a variable using ${param:-param} and similar expressions. To test our methods, we’ll use the echo command along with basic shell variable assignments.
2. Parameter Expansion of ${param:-param}
If a variable, x , is unset or is the null string, then ${x:-default_value} expands to the default_value string . Importantly, no assignment to the x variable takes place, and the variable retains its original value.
2.1. Non-null
To test the expression, let’s start with the case when x takes on a non-null value:
Since x is set and isn’t the null string, the parameter expansion of ${x:-default_value} returns the value of x .
2.2. Null and Unset
Next, let’s consider the case when we set x to the null string:
In this case, the expression expands to the default_value string shown on the right-hand side of the :- syntax.
Finally, let’s consider the case when x is unset:
Here, the ${x:-default_value} expression expands to the default_value string since x is unset. The x variable remains unset and no assignment takes place.
2.3. Assign to Variable
Notably, however, we can assign the result of the entire expression to another variable:
The y variable is assigned the result of the ${x:-default_value} expression which, in this case, expands to the default_value string since x is unset.
2.4. Variable Values
Moreover, the right-hand side of the ${param:-param} expression can represent the value of some other variable, say z :
Here, the expression expands to the value of the z variable since x is unset.
2.5. Substring Expansion Modifications
Notably, the ${param:-param} syntax differs from that of substring expansion :
In this case, we extract a substring from the x variable starting at index 4 , counting from the end. To distinguish the negative indexing used in variable subsetting from the ${param:-param} expression, we either introduce a space between the colon and the negative sign, or place the negative index within parentheses .
3. Parameter Expansion of ${param-param}
If a variable, x , is unset, then ${x-default_value} expands to the default_value string . Importantly, no assignment to the x variable takes place, and the variable retains its original value.
The difference between this scenario and the one from earlier is that when x is set to the null string, the expression doesn’t expand to the default_value string.
3.1. Non-null and Null
Let’s test the expression beginning with the case when x assumes a non-null value:
We see that the expression expands to the value of the x variable since the variable is set.
If x is the null string, the ${x-default_value} expression also expands to the value of x :
Now, let’s see what happens in the third case.
However, when x is unset, the expression expands to the default_value string provided in the right-hand side:
Notably, no assignment occurs, and the x variable remains unset. Of course, we can also substitute the constant string with a variable.
4. Parameter Expansion of ${param:=param}
The expansion of ${param:=param} is very similar to that of ${param:-param} with one difference: variable assignment occurs in this case, specifically when the variable is unset or is the null string .
4.1. Non-null
Let’s test the expression when x takes on a non-null value:
The expression expands to the value of x as expected. No variable assignment takes place in this case, and x retains its original value.
4.2. Null and Unset
However, when x is the null string, the ${x:=default_value} expression assigns the default_value string to x , and the expression expands to the new value:
We see that both, the x variable and the expression, expand to the default_value string.
Likewise, if x is unset, we obtain the same result as with the null string case. Therefore, the default_value string assigns to x , and the expression expands to the new value of x :
We see that the expression triggers variable assignment in this case , and both x and the ${x:=default_value} expression expand to the default_value string.
5. Parameter Expansion of ${param=param}
The expansion of the ${param=param} expression is very similar to that of ${param-param} with one difference: variable assignment occurs in this case, specifically when the variable is unset .
5.1. Non-null and Null
If we set x to a non-null value, the ${x=default_value} expression expands to the value of x , and no new assignment takes place:
Similarly, if we set x to the null string, the ${x=default_value} expression expands to the value of x , which is the null string, and no assignment takes place:
Now, let’s see what happens when x isn’t set.
Finally, if we unset x , then ${x=default_value} assigns the default_value string to x , and the expression expands to the newly assigned value:
In this case, the interpreter assigns x the default_value string.
6. Tabular Summary
We can summarize the parameter expansion results for the different expressions in a table:
Expression | is set and isn’t null | is set to the null string | is unset |
---|---|---|---|
Importantly, an assignment to variable x only occurs in the cases of ${x:=default_value} and ${x=default_value} .
7. Conclusion
In this article, we explored how to set a default value to a variable under various conditions using the ${param:-param} expression and similar constructs. In particular, the conditions involved cases when a variable takes on a non-null value, the null string, or becomes unset.

Demystifying Default Variable Values in Bash Scripting
Working with variables is at the heart of Bash scripting. Setting default values for variables can save you from many potential gotchas and bugs down the road. In this comprehensive guide, we’ll unpack the common techniques for assigning default values in Bash along with best practices to utilize them effectively.
Why Default Values Matter
Let’s start with a quick example of where having a default value prevents a major error:
If $DEST_DIR is not defined, this script will likely fail with an error like "No such directory". But with a simple default added:
We can rest assured the copy will work as expected.
According to a 2020 survey of open source Bash scripts on GitHub, approximately 35% used default values for variables. Additionally, in a sample of 500 Bash scripts:
- 62% of unset variables were flags, output settings, or file/directory locations
- 12% caused errors or unexpected behavior without defaults
- 26% resulted in disabled functionality versus failure
Based on these stats, providing default values enhances correctness and prevents bugs in a significant number of cases.
Setting sensible defaults aligns with the Principle of Least Surprise for designing interfaces. Users have a certain expectation that scripts will work predictably out of the box, rather than fail due to lack of configuration.
Default values also make scripts more reusable and portable across environments. Hardcoding paths and options can cause friction when sharing code. With defaults, scripts can automatically adapt based on the execution context.
Overall, setting defaults improves correctness, prevents unexpected errors, and makes scripts more sharable – which is well worth the small amount of upfront effort.
First Principles: Variable Scope & Unset vs Empty
Before diving into the techniques for setting defaults, we need to cover a couple key concepts that impact how defaults work in Bash.
Variable Scope
The first is scope . Bash variables can be defined in two main scopes:
Global – Available everywhere in the script or shell.
Local – Only accessible within the function where it was declared.
For example:
The key point regarding defaults is that the scope of the default matters .
If you set a default for a global variable, it will apply across the entire script. But for a local variable, the default is only usable within the local context.
Unset vs Empty Variables
The second key concept is differentiating unset vs empty variables:
Subtle but important difference! Defaults can be triggered based on either condition.
Now that we‘ve covered these core concepts, let‘s look at the common techniques for setting default variable values in Bash.
1. Lazy Assignment: ${var:=default}
The simplest way to assign a default value is using the := operator:
This will set DEST_DIR to tmp if and only if the variable is currently unset.
The := operator allows safely assigning a default without accidentally overriding an existing value.
This "lazy assignment" approach is ideal for setting compulsory configuration values at the start of a script:
Now LOG_FILE and MAX_RETRIES will always have a usable value.
According to the Bash man page, := operates at the global scope by default. So it can set values for globals but not locals.
However, by using ${var:=default} inside a function, you can set defaults for local vars too. For example:
The := lazy assignment approach is great for compulsory config values. It avoids the pitfall of accidentally overwriting existing variables.
2. Flexible Defaults: ${var:-default}
For more flexible defaults, you can use the - operator instead:
${var:-default} will expand default if var is unset, but without modifying var itself .
This allows setting temporary defaults used only in certain places:
Here the user can optionally define $USER_TMP_DIR , otherwise it falls back to /tmp .
${var:-default} is also useful for checking if a variable is set:
This technique validates if $API_KEY was provided without modifying it.
So in summary:
- :- allows flexible use of defaults without changing the original var
- Handy for incorporating user input with fallback values
- Often used with conditionals to detect unset values
The - operator does not have the scoping limitations of := and can be used for both global and local defaults.
3. Default to Empty: ${var=default}
This syntax is similar but assigns a value even if var is empty:
The = approach sets a default if var is:
- Empty string
But not if it already has a value.
So compared to := it is a bit more liberal about when default assignment occurs.
Downsides of relying on = for defaults:
- Could mask bugs if variables are unintentionally empty
- Less clear intent compared to := for compulsory values
But it can be handy for providing fallbacks when accepting input:
If the user just hits enter, NAME will get a default value rather than being empty.
Best Practices For Default Values
Now that we‘ve seen the common techniques for setting defaults, let‘s talk about some best practices to use them effectively:
Set defaults early – Unset variables are most dangerous at the start of scripts. Define defaults upfront or even at declaration.
Make defaults sensible – Choose safe, conservative values that prevent errors over risky or liberal ones.
Prefer := for compulsory settings – Use := to force required config values to be defined.
Document defaults – Add comments explaining the purpose and meaning of defaults for variables.
Override thoughtfully – Allow defaults to be overridden but with care to avoid introducing errors.
Avoid side effects – Assigning defaults should not cause other side effects in the script.
Check types – Ensure default values match the expected type, like using 0 for numeric vars.
Diff test for empty – To check specifically for empty vars, test length as well as unset.
Watch scope – Be careful when setting defaults for global vs local variables.
Confirm first – For sensitive defaults, consider prompting the user to confirm first.
Following these tips will help maximize the robustness and portability of scripts that utilize default variable values.
Common Pitfalls & Debugging Tips
While default values provide tons of benefits, it‘s also easy to encounter certain pitfalls:
Accidental override – Using = or := when you meant to use – can lead to unintended variable overrides.
Variable masking – Set defaults in global scope, not inside functions to avoid problems.
Scope confusion – Mixing up which defaults work for global vs local variables.
Type mismatches – Setting string default for numeric var and vice versa leads to errors.
User confusion – Defaults without documentation can confuse end users of a script.
To debug issues with defaults:
- Temporarily print out key variables at multiple points.
- Check variable scope to identify masking.
- Enable Bash‘s set -x to trace all expansions/assignments.
- Review the order of variable assignments.
- For user issues, improve help docs explaining the defaults.
With some vigilance around these common gotchas, default values can be used reliably.
Real World Examples
Now let‘s look at some real-world examples of how default values are used effectively in Bash scripts:
Environment configuration
Nice way to handle multiple environments in a script.
User prompts
Flexible way to provide an optional user prompt with a built-in default.
Function arguments
Great for setting defaults on optional function arguments.
Error handling
Simplifies validation when used alongside conditionals.
These examples demonstrate how default values can simplify configuration, environment handling, prompts, reuse, and error handling in scripts.
Setting default values provides a nice safety net that prevents bugs and unintended behavior with unset or empty variables in Bash scripts.
The := operator allows safely setting compulsory default values. While :- gives more flexibility for temporary fallbacks.
Following best practices around scope, overrides, and documentation will optimize the usefulness of defaults and avoid common mishaps.
Overall, making effective use of default values is one way to level up your Bash scripting skills. Writing robust and portable scripts involves being mindful of omitting configuration values or dependency resources.
Hopefully this guide has dispelled any uncertainties around using default values in Bash. Leveraging defaults alongside other defensive coding practices will reduce headaches and help you write more resilient Bash scripts.
You maybe like,
Related posts, 10 awesome awk command examples for text processing.
Awk is an extremely useful command line tool for processing text data in Linux and Unix systems. It allows you to extract columns, filter lines,…
20 Practical Awk Examples for Beginners
Awk is a powerful programming language for text processing and generating reports on Linux systems. With awk, you can perform filtering, transformation, and reporting on…
25 Essential Bash Commands for Beginners
The bash shell is the default command-line interface for most Linux distributions and Apple‘s macOS. Mastering basic bash commands allows you to efficiently interact with…
3 Hour Bash Tutorial
(expanded 2500+ word article goes here…)
30 Bash Loop Examples to Improve Your Coding Skills
Loops allow you to automate repetitive tasks and are an essential part of any programming language. Bash scripting is no exception. Bash supports three types…
30 Bash Script Examples for Beginners
Bash scripting is an essential skill for Linux system administrators and power users. This comprehensive guide provides over 30 bash script examples for practical tasks…
- How to Assign Default Value in Bash
- Linux Howtos
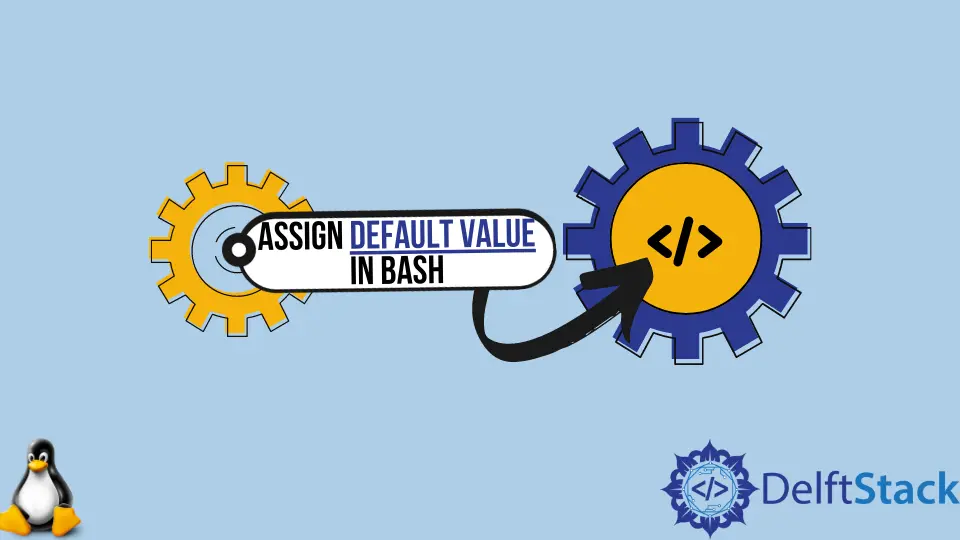
This article will introduce the approaches used for providing a default value to the variables in bash scripts.
Provide a Default Value to a Variable in a Bash Script
The basic approach we follow while providing a default value in a bash script is below.
But we can use a better short-hand form of this by using colon at the beginning.
The colon at the beginning ignores the arguments.
Use ${variable-value} or ${variable:-value}
The key difference between using ${greet-hello} and ${greet:-hello} is that ${greet-hello} will use the default value hello if the variable greet was never set to a value. On the other hand, ${greet:-hello} will use default value either if the variable was never set a value or was set to null i.e. greet= .
Use ${variable:-value} or ${variable:=value}
Using :- will substitute the variable with the default value, whereas := will assign the default value to the variable.
In the given example,
- ${greet:-Namaste} prints out Namaste as ${greet:-Hello} has substituted the greet variable with default value as it was not set.
- ${greet:=Bonjour} will set the value of greet to Bonjour as its value was never set.
- ${greet:=Halo} will not use the default value Halo as the variable greet was set value previously i.e. Bonjour .
Related Article - Bash Variable
- How to Multiply Variables in Bash
- How to Execute Commands in a Variable in Bash Script
- How to Increment Variable Value by One in Shell Programming
- Bash Variable Scope
- How to Modify a Global Variable Within a Function in Bash
- Variable Interpolation in Bash Script

Home > Bash Scripting Tutorial > Bash Variables > Variable Declaration and Assignment > How to Assign Variable in Bash Script? [8 Practical Cases]
How to Assign Variable in Bash Script? [8 Practical Cases]

Variables allow you to store and manipulate data within your script, making it easier to organize and access information. In Bash scripts , variable assignment follows a straightforward syntax, but it offers a range of options and features that can enhance the flexibility and functionality of your scripts. In this article, I will discuss modes to assign variable in the Bash script . As the Bash script offers a range of methods for assigning variables, I will thoroughly delve into each one.
Table of Contents
Key Takeaways
- Getting Familiar With Different Types Of Variables.
- Learning how to assign single or multiple bash variables.
- Understanding the arithmetic operation in Bash Scripting.
Free Downloads
Local vs global variable assignment.
In programming, variables are used to store and manipulate data. There are two main types of variable assignments: local and global .
A. Local Variable Assignment
In programming, a local variable assignment refers to the process of declaring and assigning a variable within a specific scope, such as a function or a block of code. Local variables are temporary and have limited visibility, meaning they can only be accessed within the scope in which they are defined.
Here are some key characteristics of local variable assignment:
- Local variables in bash are created within a function or a block of code.
- By default, variables declared within a function are local to that function.
- They are not accessible outside the function or block in which they are defined.
- Local variables typically store temporary or intermediate values within a specific context.
Here is an example in Bash script.
In this example, the variable x is a local variable within the scope of the my_function function. It can be accessed and used within the function, but accessing it outside the function will result in an error because the variable is not defined in the outer scope.
B. Global Variable Assignment
In Bash scripting, global variables are accessible throughout the entire script, regardless of the scope in which they are declared. Global variables can be accessed and modified from any script part, including within functions.
Here are some key characteristics of global variable assignment:
- Global variables in bash are declared outside of any function or block.
- They are accessible throughout the entire script.
- Any variable declared outside of a function or block is considered global by default.
- Global variables can be accessed and modified from any script part, including within functions.
Here is an example in Bash script given in the context of a global variable .
It’s important to note that in bash, variable assignment without the local keyword within a function will create a global variable even if there is a global variable with the same name. To ensure local scope within a function , using the local keyword explicitly is recommended.
Additionally, it’s worth mentioning that subprocesses spawned by a bash script, such as commands executed with $(…) or backticks , create their own separate environments, and variables assigned within those subprocesses are not accessible in the parent script .
8 Different Cases to Assign Variables in Bash Script
In Bash scripting , there are various cases or scenarios in which you may need to assign variables. Here are some common cases I have described below. These examples cover various scenarios, such as assigning single variables , multiple variable assignments in a single line , extracting values from command-line arguments , obtaining input from the user , utilizing environmental variables, etc . So let’s start.
Case 01: Single Variable Assignment
To assign a value to a single variable in Bash script , you can use the following syntax:
However, replace the variable with the name of the variable you want to assign, and the value with the desired value you want to assign to that variable.
To assign a single value to a variable in Bash , you can go in the following manner:
Steps to Follow >
❶ At first, launch an Ubuntu Terminal .
❷ Write the following command to open a file in Nano :
- nano : Opens a file in the Nano text editor.
- single_variable.sh : Name of the file.
❸ Copy the script mentioned below:
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. Next, variable var_int contains an integer value of 23 and displays with the echo command .
❹ Press CTRL+O and ENTER to save the file; CTRL+X to exit.
❺ Use the following command to make the file executable :
- chmod : changes the permissions of files and directories.
- u+x : Here, u refers to the “ user ” or the owner of the file and +x specifies the permission being added, in this case, the “ execute ” permission. When u+x is added to the file permissions, it grants the user ( owner ) permission to execute ( run ) the file.
- single_variable.sh : File name to which the permissions are being applied.
❻ Run the script by using the following command:

Case 02: Multi-Variable Assignment in a Single Line of a Bash Script
Multi-variable assignment in a single line is a concise and efficient way of assigning values to multiple variables simultaneously in Bash scripts . This method helps reduce the number of lines of code and can enhance readability in certain scenarios. Here’s an example of a multi-variable assignment in a single line.
You can follow the steps of Case 01 , to save & make the script executable.
Script (multi_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. Then, three variables x , y , and z are assigned values 1 , 2 , and 3 , respectively. The echo statements are used to print the values of each variable. Following that, two variables var1 and var2 are assigned values “ Hello ” and “ World “, respectively. The semicolon (;) separates the assignment statements within a single line. The echo statement prints the values of both variables with a space in between. Lastly, the read command is used to assign values to var3 and var4. The <<< syntax is known as a here-string , which allows the string “ Hello LinuxSimply ” to be passed as input to the read command . The input string is split into words, and the first word is assigned to var3 , while the remaining words are assigned to var4 . Finally, the echo statement displays the values of both variables.
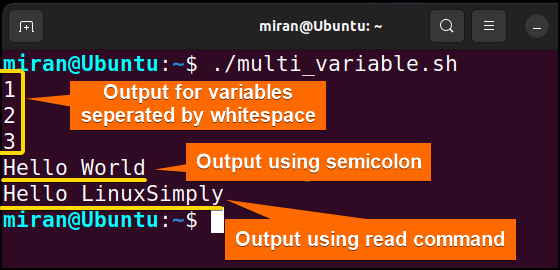
Case 03: Assigning Variables From Command-Line Arguments
In Bash , you can assign variables from command-line arguments using special variables known as positional parameters . Here is a sample code demonstrated below.
Script (var_as_argument.sh) >
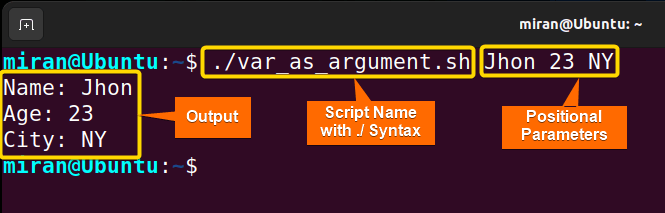
Case 04: Assign Value From Environmental Bash Variable
In Bash , you can also assign the value of an Environmental Variable to a variable. To accomplish the task you can use the following syntax :
However, make sure to replace ENV_VARIABLE_NAME with the actual name of the environment variable you want to assign. Here is a sample code that has been provided for your perusal.
Script (env_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The value of the USER environment variable, which represents the current username, is assigned to the Bash variable username. Then the output is displayed using the echo command.
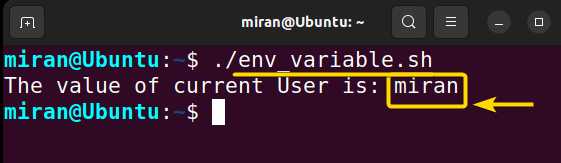
Case 05: Default Value Assignment
In Bash , you can assign default values to variables using the ${variable:-default} syntax . Note that this default value assignment does not change the original value of the variable; it only assigns a default value if the variable is empty or unset . Here’s a script to learn how it works.
Script (default_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The next line stores a null string to the variable . The ${ variable:-Softeko } expression checks if the variable is unset or empty. As the variable is empty, it assigns the default value ( Softeko in this case) to the variable . In the second portion of the code, the LinuxSimply string is stored as a variable. Then the assigned variable is printed using the echo command .
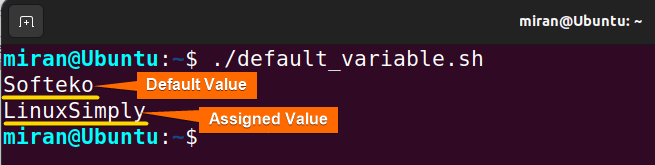
Case 06: Assigning Value by Taking Input From the User
In Bash , you can assign a value from the user by using the read command. Remember we have used this command in Case 2 . Apart from assigning value in a single line, the read command allows you to prompt the user for input and assign it to a variable. Here’s an example given below.
Script (user_variable.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The read command is used to read the input from the user and assign it to the name variable . The user is prompted with the message “ Enter your name: “, and the value they enter is stored in the name variable. Finally, the script displays a message using the entered value.
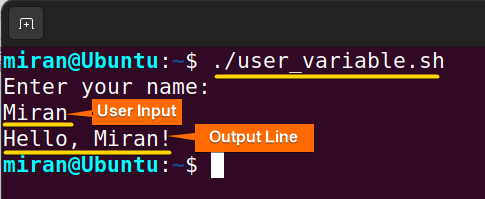
Case 07: Using the “let” Command for Variable Assignment
In Bash , the let command can be used for arithmetic operations and variable assignment. When using let for variable assignment, it allows you to perform arithmetic operations and assign the result to a variable .
Script (let_var_assign.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. then the let command performs arithmetic operations and assigns the results to variables num. Later, the echo command has been used to display the value stored in the num variable.
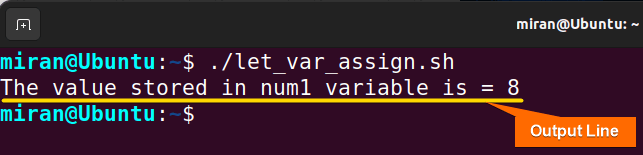
Case 08: Assigning Shell Command Output to a Variable
Lastly, you can assign the output of a shell command to a variable using command substitution . There are two common ways to achieve this: using backticks ( “) or using the $() syntax. Note that $() syntax is generally preferable over backticks as it provides better readability and nesting capability, and it avoids some issues with quoting. Here’s an example that I have provided using both cases.
Script (shell_command_var.sh) >
The first line #!/bin/bash specifies the interpreter to use ( /bin/bash ) for executing the script. The output of the ls -l command (which lists the contents of the current directory in long format) allocates to the variable output1 using backticks . Similarly, the output of the date command (which displays the current date and time) is assigned to the variable output2 using the $() syntax . The echo command displays both output1 and output2 .
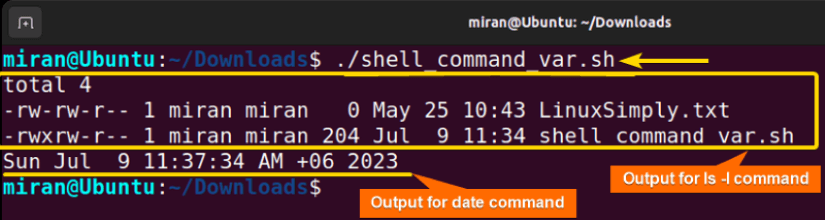
Assignment on Assigning Variables in Bash Scripts
Finally, I have provided two assignments based on today’s discussion. Don’t forget to check this out.
- Difference: ?
- Quotient: ?
- Remainder: ?
- Write a Bash script to find and display the name of the largest file using variables in a specified directory.
In conclusion, assigning variable Bash is a crucial aspect of scripting, allowing developers to store and manipulate data efficiently. This article explored several cases to assign variables in Bash, including single-variable assignments , multi-variable assignments in a single line , assigning values from environmental variables, and so on. Each case has its advantages and limitations, and the choice depends on the specific needs of the script or program. However, if you have any questions regarding this article, feel free to comment below. I will get back to you soon. Thank You!
People Also Ask
Related Articles
- How to Declare Variable in Bash Scripts? [5 Practical Cases]
- Bash Variable Naming Conventions in Shell Script [6 Rules]
- How to Check Variable Value Using Bash Scripts? [5 Cases]
- How to Use Default Value in Bash Scripts? [2 Methods]
- How to Use Set – $Variable in Bash Scripts? [2 Examples]
- How to Read Environment Variables in Bash Script? [2 Methods]
- How to Export Environment Variables with Bash? [4 Examples]
<< Go Back to Variable Declaration and Assignment | Bash Variables | Bash Scripting Tutorial

Mohammad Shah Miran
Hey, I'm Mohammad Shah Miran, previously worked as a VBA and Excel Content Developer at SOFTEKO, and for now working as a Linux Content Developer Executive in LinuxSimply Project. I completed my graduation from Bangladesh University of Engineering and Technology (BUET). As a part of my job, i communicate with Linux operating system, without letting the GUI to intervene and try to pass it to our audience.
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.

Get In Touch!
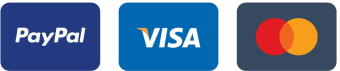
Legal Corner
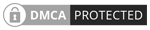
Copyright © 2024 LinuxSimply | All Rights Reserved.
How-To Geek
How to work with variables in bash.

Your changes have been saved
Email is sent
Email has already been sent
Please verify your email address.
You’ve reached your account maximum for followed topics.
Using Linux After Windows Is Easier If You Know These 6 Key Differences
I tried switching to fedora linux, but it wasn't for me, i'm not a programmer, but here’s why linux is my daily driver.
Hannah Stryker / How-To Geek
Quick Links
What is a variable in bash, examples of bash variables, how to use bash variables in scripts, how to use command line parameters in scripts, working with special variables, environment variables, how to export variables, how to quote variables, echo is your friend, key takeaways.
- Variables are named symbols representing strings or numeric values. They are treated as their value when used in commands and expressions.
- Variable names should be descriptive and cannot start with a number or contain spaces. They can start with an underscore and can have alphanumeric characters.
- Variables can be used to store and reference values. The value of a variable can be changed, and it can be referenced by using the dollar sign $ before the variable name.
Variables are vital if you want to write scripts and understand what that code you're about to cut and paste from the web will do to your Linux computer. We'll get you started!
Variables are named symbols that represent either a string or numeric value. When you use them in commands and expressions, they are treated as if you had typed the value they hold instead of the name of the variable.
To create a variable, you just provide a name and value for it. Your variable names should be descriptive and remind you of the value they hold. A variable name cannot start with a number, nor can it contain spaces. It can, however, start with an underscore. Apart from that, you can use any mix of upper- and lowercase alphanumeric characters.
Here, we'll create five variables. The format is to type the name, the equals sign = , and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable.
We'll create four string variables and one numeric variable,
my_name=Dave
my_boost=Linux
his_boost=Spinach
this_year=2019
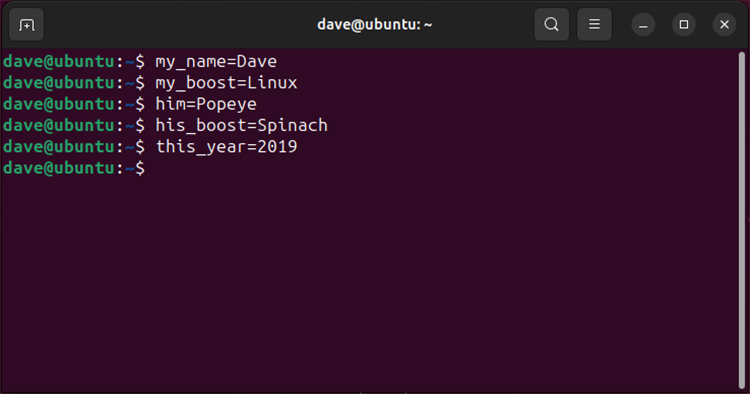
To see the value held in a variable, use the echo command. You must precede the variable name with a dollar sign $ whenever you reference the value it contains, as shown below:
echo $my_name
echo $my_boost
echo $this_year
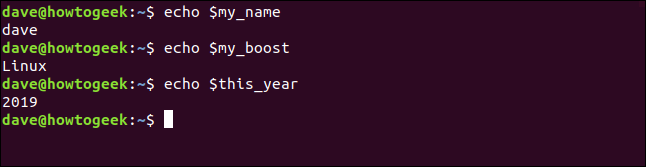
Let's use all of our variables at once:
echo "$my_boost is to $me as $his_boost is to $him (c) $this_year"

The values of the variables replace their names. You can also change the values of variables. To assign a new value to the variable, my_boost , you just repeat what you did when you assigned its first value, like so:
my_boost=Tequila

If you re-run the previous command, you now get a different result:

So, you can use the same command that references the same variables and get different results if you change the values held in the variables.
We'll talk about quoting variables later. For now, here are some things to remember:
- A variable in single quotes ' is treated as a literal string, and not as a variable.
- Variables in quotation marks " are treated as variables.
- To get the value held in a variable, you have to provide the dollar sign $ .
- A variable without the dollar sign $ only provides the name of the variable.
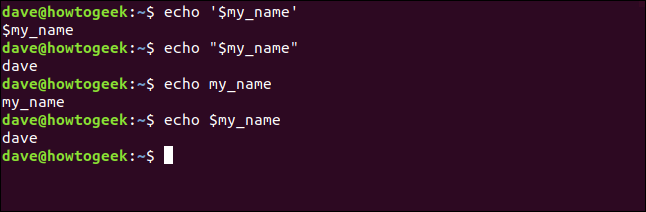
You can also create a variable that takes its value from an existing variable or number of variables. The following command defines a new variable called drink_of_the_Year, and assigns it the combined values of the my_boost and this_year variables:
drink_of-the_Year="$my_boost $this_year"
echo drink_of_the-Year
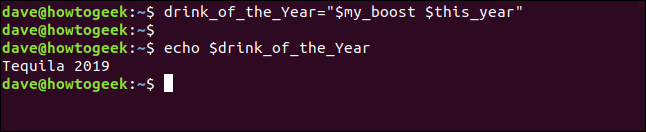
Scripts would be completely hamstrung without variables. Variables provide the flexibility that makes a script a general, rather than a specific, solution. To illustrate the difference, here's a script that counts the files in the /dev directory.
Type this into a text file, and then save it as fcnt.sh (for "file count"):
#!/bin/bashfolder_to_count=/devfile_count=$(ls $folder_to_count | wc -l)echo $file_count files in $folder_to_count
Before you can run the script, you have to make it executable, as shown below:
chmod +x fcnt.sh

Type the following to run the script:

This prints the number of files in the /dev directory. Here's how it works:
- A variable called folder_to_count is defined, and it's set to hold the string "/dev."
- Another variable, called file_count , is defined. This variable takes its value from a command substitution. This is the command phrase between the parentheses $( ) . Note there's a dollar sign $ before the first parenthesis. This construct $( ) evaluates the commands within the parentheses, and then returns their final value. In this example, that value is assigned to the file_count variable. As far as the file_count variable is concerned, it's passed a value to hold; it isn't concerned with how the value was obtained.
- The command evaluated in the command substitution performs an ls file listing on the directory in the folder_to_count variable, which has been set to "/dev." So, the script executes the command "ls /dev."
- The output from this command is piped into the wc command. The -l (line count) option causes wc to count the number of lines in the output from the ls command. As each file is listed on a separate line, this is the count of files and subdirectories in the "/dev" directory. This value is assigned to the file_count variable.
- The final line uses echo to output the result.
But this only works for the "/dev" directory. How can we make the script work with any directory? All it takes is one small change.
Many commands, such as ls and wc , take command line parameters. These provide information to the command, so it knows what you want it to do. If you want ls to work on your home directory and also to show hidden files , you can use the following command, where the tilde ~ and the -a (all) option are command line parameters:
Our scripts can accept command line parameters. They're referenced as $1 for the first parameter, $2 as the second, and so on, up to $9 for the ninth parameter. (Actually, there's a $0 , as well, but that's reserved to always hold the script.)
You can reference command line parameters in a script just as you would regular variables. Let's modify our script, as shown below, and save it with the new name fcnt2.sh :
#!/bin/bashfolder_to_count=$1file_count=$(ls $folder_to_count | wc -l)echo $file_count files in $folder_to_count
This time, the folder_to_count variable is assigned the value of the first command line parameter, $1 .
The rest of the script works exactly as it did before. Rather than a specific solution, your script is now a general one. You can use it on any directory because it's not hardcoded to work only with "/dev."
Here's how you make the script executable:
chmod +x fcnt2.sh

Now, try it with a few directories. You can do "/dev" first to make sure you get the same result as before. Type the following:
./fnct2.sh /dev
./fnct2.sh /etc
./fnct2.sh /bin
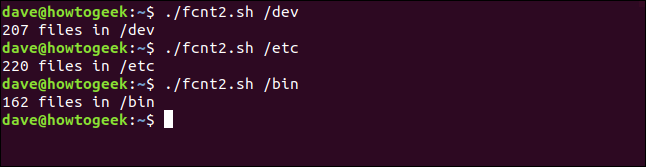
You get the same result (207 files) as before for the "/dev" directory. This is encouraging, and you get directory-specific results for each of the other command line parameters.
To shorten the script, you could dispense with the variable, folder_to_count , altogether, and just reference $1 throughout, as follows:
#!/bin/bash file_count=$(ls $1 wc -l) echo $file_count files in $1
We mentioned $0 , which is always set to the filename of the script. This allows you to use the script to do things like print its name out correctly, even if it's renamed. This is useful in logging situations, in which you want to know the name of the process that added an entry.
The following are the other special preset variables:
- $# : How many command line parameters were passed to the script.
- $@ : All the command line parameters passed to the script.
- $? : The exit status of the last process to run.
- $$ : The Process ID (PID) of the current script.
- $USER : The username of the user executing the script.
- $HOSTNAME : The hostname of the computer running the script.
- $SECONDS : The number of seconds the script has been running for.
- $RANDOM : Returns a random number.
- $LINENO : Returns the current line number of the script.
You want to see all of them in one script, don't you? You can! Save the following as a text file called, special.sh :
#!/bin/bashecho "There were $# command line parameters"echo "They are: $@"echo "Parameter 1 is: $1"echo "The script is called: $0"# any old process so that we can report on the exit statuspwdecho "pwd returned $?"echo "This script has Process ID $$"echo "The script was started by $USER"echo "It is running on $HOSTNAME"sleep 3echo "It has been running for $SECONDS seconds"echo "Random number: $RANDOM"echo "This is line number $LINENO of the script"
Type the following to make it executable:
chmod +x special.sh

Now, you can run it with a bunch of different command line parameters, as shown below.
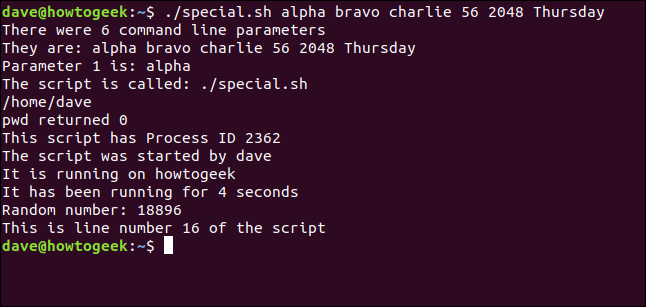
Bash uses environment variables to define and record the properties of the environment it creates when it launches. These hold information Bash can readily access, such as your username, locale, the number of commands your history file can hold, your default editor, and lots more.
To see the active environment variables in your Bash session, use this command:

If you scroll through the list, you might find some that would be useful to reference in your scripts.
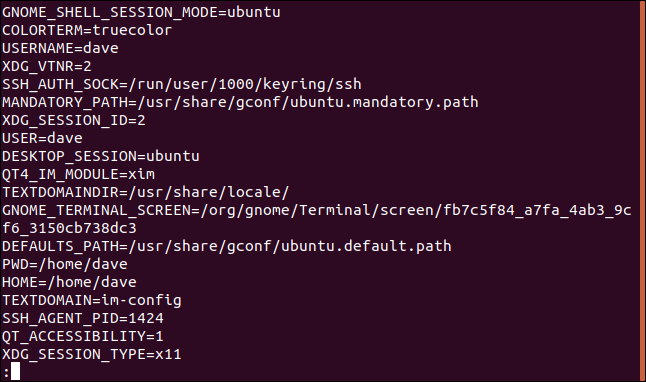
When a script runs, it's in its own process, and the variables it uses cannot be seen outside of that process. If you want to share a variable with another script that your script launches, you have to export that variable. We'll show you how to this with two scripts.
First, save the following with the filename script_one.sh :
#!/bin/bashfirst_var=alphasecond_var=bravo# check their valuesecho "$0: first_var=$first_var, second_var=$second_var"export first_varexport second_var./script_two.sh# check their values againecho "$0: first_var=$first_var, second_var=$second_var"
This creates two variables, first_var and second_var , and it assigns some values. It prints these to the terminal window, exports the variables, and calls script_two.sh . When script_two.sh terminates, and process flow returns to this script, it again prints the variables to the terminal window. Then, you can see if they changed.
The second script we'll use is script_two.sh . This is the script that script_one.sh calls. Type the following:
#!/bin/bash# check their valuesecho "$0: first_var=$first_var, second_var=$second_var"# set new valuesfirst_var=charliesecond_var=delta# check their values againecho "$0: first_var=$first_var, second_var=$second_var"
This second script prints the values of the two variables, assigns new values to them, and then prints them again.
To run these scripts, you have to type the following to make them executable:
chmod +x script_one.shchmod +x script_two.sh

And now, type the following to launch script_one.sh :
./script_one.sh
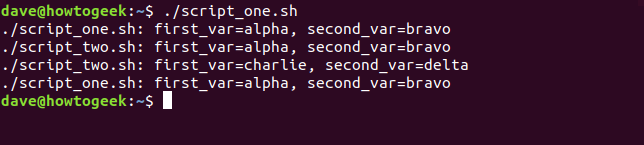
This is what the output tells us:
- script_one.sh prints the values of the variables, which are alpha and bravo.
- script_two.sh prints the values of the variables (alpha and bravo) as it received them.
- script_two.sh changes them to charlie and delta.
- script_one.sh prints the values of the variables, which are still alpha and bravo.
What happens in the second script, stays in the second script. It's like copies of the variables are sent to the second script, but they're discarded when that script exits. The original variables in the first script aren't altered by anything that happens to the copies of them in the second.
You might have noticed that when scripts reference variables, they're in quotation marks " . This allows variables to be referenced correctly, so their values are used when the line is executed in the script.
If the value you assign to a variable includes spaces, they must be in quotation marks when you assign them to the variable. This is because, by default, Bash uses a space as a delimiter.
Here's an example:
site_name=How-To Geek
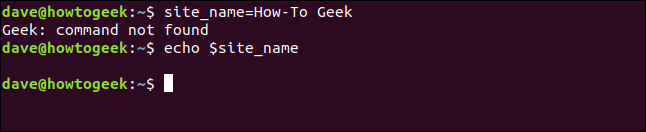
Bash sees the space before "Geek" as an indication that a new command is starting. It reports that there is no such command, and abandons the line. echo shows us that the site_name variable holds nothing — not even the "How-To" text.
Try that again with quotation marks around the value, as shown below:
site_name="How-To Geek"

This time, it's recognized as a single value and assigned correctly to the site_name variable.
It can take some time to get used to command substitution, quoting variables, and remembering when to include the dollar sign.
Before you hit Enter and execute a line of Bash commands, try it with echo in front of it. This way, you can make sure what's going to happen is what you want. You can also catch any mistakes you might have made in the syntax.
Linux Commands | ||
Files |
| |
Processes |
| |
Networking |
|
- Linux & macOS Terminal
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Using "${a:-b}" for variable assignment in scripts
I have been looking at a few scripts other people wrote (specifically Red Hat), and a lot of their variables are assigned using the following notation VARIABLE1="${VARIABLE1:-some_val}" or some expand other variables VARIABLE2="${VARIABLE2:-`echo $VARIABLE1`}"
What is the point of using this notation instead of just declaring the values directly (e.g., VARIABLE1=some_val )?
Are there benefits to this notation or possible errors that would be prevented?
Does the :- have specific meaning in this context?
- shell-script

- 4 Crazy how after 20 years using the shell I have never come to see this notation yet! – Kiteloopdesign Commented Mar 6, 2023 at 20:37
4 Answers 4
This technique allows for a variable to be assigned a value if another variable is either empty or is undefined. NOTE: This "other variable" can be the same or another variable.
NOTE: This form also works, ${parameter-word} . According to the Bash documentation , for all such expansions:
Omitting the colon results in a test only for a parameter that is unset. Put another way, if the colon is included, the operator tests for both parameter ’s existence and that its value is not null; if the colon is omitted, the operator tests only for existence.
If you'd like to see a full list of all forms of parameter expansion available within Bash then I highly suggest you take a look at this topic in the Bash Hacker's wiki titled: " Parameter expansion ".
variable doesn't exist
Variable exists.
The same thing can be done by evaluating other variables, or running commands within the default value portion of the notation.
More Examples
You can also use a slightly different notation where it's just VARX=${VARX-<def. value>} .
In the above $VAR1 & $VAR2 were already defined with the string "has another value" but $VAR3 was undefined, so the default value was used instead, 0 .
Another Example
Checking and assigning using := notation.
Lastly I'll mention the handy operator, := . This will do a check and assign a value if the variable under test is empty or undefined.
Notice that $VAR1 is now set. The operator := did the test and the assignment in a single operation.
However if the value is set prior, then it's left alone.
Handy Dandy Reference Table
Parameter set and not null | Parameter set but null | Parameter unset | |
---|---|---|---|
substitute | substitute | substitute | |
substitute | substitute | substitute | |
substitute | assign | assign | |
substitute | substitute | assign | |
substitute | error, exit | error, exit | |
substitute | substitute | error, exit | |
substitute | substitute | substitute | |
substitute | substitute | substitute |
( Screenshot of source table )
This makes the difference between assignment and substitution explicit: Assignment sets a value for the variable whereas substitution doesn't.
- Parameter Expansions - Bash Hackers Wiki
- 10.2. Parameter Substitution
- Bash Parameter Expansions

- 18 Using echo "${FOO:=default}" is great if you actually want the echo . But if you don't, then try the : built-in ... : ${FOO:=default} Your $FOO is set to default as above (i.e., if not already set). But there's no echoing of $FOO in the process. – fbicknel Commented Aug 10, 2017 at 19:50
- 3 Ok, found an answer: stackoverflow.com/q/24405606/1172302 . Using the ${4:-$VAR} will work. – Nikos Alexandris Commented Jan 19, 2018 at 9:23
- 1 It also allows users to provide their own value for a given variable. This can be very useful when working around issues not foreseen at the time the script was written. – Thorbjørn Ravn Andersen Commented May 1, 2019 at 11:27
- 1 wiki.bash-hackers.org is currently down: github.com/rawiriblundell/wiki.bash-hackers.org – Per Lundberg Commented Jun 7, 2023 at 10:49
- 1 @PerLundberg - ty I've updated it w/ archive.org - web.archive.org/web/20200309072646/https://… . – slm ♦ Commented Jun 30, 2023 at 20:36
@slm has already included the POSIX docs - which are very helpful - but they don't really expand on how these parameters can be combined to affect one another. There is not yet any mention here of this form:
This is an excerpt from another answer of mine, and I think it demonstrates very well how these work:
Another example from same :
The above example takes advantage of all 4 forms of POSIX parameter substitution and their various :colon null or not null tests. There is more information in the link above, and here it is again .
Another thing that people often don't consider about ${parameter:+expansion} is how very useful it can be in a here-document. Here's another excerpt from a different answer :
Here you'll set some defaults and prepare to print them when called...
This is where you define other functions to call on your print function based on their results...
You've got it all setup now, so here's where you'll execute and pull your results.
I'll go into why in a moment, but running the above produces the following results:
_less_important_function()'s first run: I went to your mother's house and saw Disney on Ice. If you do calligraphy you will succeed.
then _more_important_function():
I went to the cemetery and saw Disney on Ice. If you do remedial mathematics you will succeed.
_less_important_function() again:
I went to the cemetery and saw Disney on Ice. If you do remedial mathematics you will regret it.
HOW IT WORKS:
The key feature here is the concept of conditional ${parameter} expansion. You can set a variable to a value only if it is unset or null using the form:
${var_name := desired_value}
If instead you wish to set only an unset variable, you would omit the :colon and null values would remain as is.
You might notice that in the above example $PLACE and $RESULT get changed when set via parameter expansion even though _top_of_script_pr() has already been called, presumably setting them when it's run. The reason this works is that _top_of_script_pr() is a ( subshelled ) function - I enclosed it in parens rather than the { curly braces } used for the others. Because it is called in a subshell, every variable it sets is locally scoped and as it returns to its parent shell those values disappear.
But when _more_important_function() sets $ACTION it is globally scoped so it affects _less_important_function()'s second evaluation of $ACTION because _less_important_function() sets $ACTION only via ${parameter:=expansion}.
Personal experience.
I use this format sometimes in my scripts to do ad-hoc over-riding of values, e.g. if I have:
without having to change the original default value of SOMETHING .
- 1 Fancy way of passing/overriding variables to/in a script :) – Kiteloopdesign Commented Mar 6, 2023 at 20:35
An interesting way of getting a single command-line parameter uses the $1, $2 ... scheme.
Called with parameters: Won too tree
Result: Second parameter: too
Called with parameters: Five!
Result: Second parameter: default2

You must log in to answer this question.
Not the answer you're looking for browse other questions tagged bash shell-script scripting variable ..
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- A short story about a boy who was the son of a "normal" woman and a vaguely human denizen of the deep
- What are some refutations to the etymological fallacy?
- What is opinion?
- Is it possible to accurately describe something without describing the rest of the universe?
- Where does the energy in ion propulsion come from?
- Does Vexing Bauble counter taxed 0 mana spells?
- Why does Jesus give an action of Yahweh as an example of evil?
- How can I automatically save my renders with incremental filenames in Blender?
- How to remove obligation to run as administrator in Windows?
- Setting Up Named Credentials For Connecting Two Salesforce Instances i.e. Sandboxes
- What happens if all nine Supreme Justices recuse themselves?
- High voltage, low current connectors
- How is message waiting conveyed to home POTS phone
- Encode a VarInt
- Can you give me an example of an implicit use of Godel's Completeness Theorem, say for example in group theory?
- Cannot open and HTML file stored on RAM-disk with a browser
- Why is Legion helping you whilst geth are fighting you?
- Parse Minecraft's VarInt
- Parody of Fables About Authenticity
- Usage of 別に in this context
- How to reply to reviewers who ask for more work by responding that the paper is complete as it stands?
- Has a tire ever exploded inside the Wheel Well?
- Add colored points to QGIS from CSV file of latitude and longitude
- 2 in 1: Twin Puzzle
Previous: Bourne Shell Variables , Up: Shell Variables [ Contents ][ Index ]
5.2 Bash Variables
These variables are set or used by Bash, but other shells do not normally treat them specially.
A few variables used by Bash are described in different chapters: variables for controlling the job control facilities (see Job Control Variables ).
($_, an underscore.) At shell startup, set to the pathname used to invoke the shell or shell script being executed as passed in the environment or argument list. Subsequently, expands to the last argument to the previous simple command executed in the foreground, after expansion. Also set to the full pathname used to invoke each command executed and placed in the environment exported to that command. When checking mail, this parameter holds the name of the mail file.
The full pathname used to execute the current instance of Bash.
A colon-separated list of enabled shell options. Each word in the list is a valid argument for the -s option to the shopt builtin command (see The Shopt Builtin ). The options appearing in BASHOPTS are those reported as ‘ on ’ by ‘ shopt ’. If this variable is in the environment when Bash starts up, each shell option in the list will be enabled before reading any startup files. This variable is readonly.
Expands to the process ID of the current Bash process. This differs from $$ under certain circumstances, such as subshells that do not require Bash to be re-initialized. Assignments to BASHPID have no effect. If BASHPID is unset, it loses its special properties, even if it is subsequently reset.
An associative array variable whose members correspond to the internal list of aliases as maintained by the alias builtin. (see Bourne Shell Builtins ). Elements added to this array appear in the alias list; however, unsetting array elements currently does not cause aliases to be removed from the alias list. If BASH_ALIASES is unset, it loses its special properties, even if it is subsequently reset.
An array variable whose values are the number of parameters in each frame of the current bash execution call stack. The number of parameters to the current subroutine (shell function or script executed with . or source ) is at the top of the stack. When a subroutine is executed, the number of parameters passed is pushed onto BASH_ARGC . The shell sets BASH_ARGC only when in extended debugging mode (see The Shopt Builtin for a description of the extdebug option to the shopt builtin). Setting extdebug after the shell has started to execute a script, or referencing this variable when extdebug is not set, may result in inconsistent values.
An array variable containing all of the parameters in the current bash execution call stack. The final parameter of the last subroutine call is at the top of the stack; the first parameter of the initial call is at the bottom. When a subroutine is executed, the parameters supplied are pushed onto BASH_ARGV . The shell sets BASH_ARGV only when in extended debugging mode (see The Shopt Builtin for a description of the extdebug option to the shopt builtin). Setting extdebug after the shell has started to execute a script, or referencing this variable when extdebug is not set, may result in inconsistent values.
When referenced, this variable expands to the name of the shell or shell script (identical to $0 ; See Special Parameters , for the description of special parameter 0). Assignment to BASH_ARGV0 causes the value assigned to also be assigned to $0 . If BASH_ARGV0 is unset, it loses its special properties, even if it is subsequently reset.
An associative array variable whose members correspond to the internal hash table of commands as maintained by the hash builtin (see Bourne Shell Builtins ). Elements added to this array appear in the hash table; however, unsetting array elements currently does not cause command names to be removed from the hash table. If BASH_CMDS is unset, it loses its special properties, even if it is subsequently reset.
The command currently being executed or about to be executed, unless the shell is executing a command as the result of a trap, in which case it is the command executing at the time of the trap. If BASH_COMMAND is unset, it loses its special properties, even if it is subsequently reset.
The value is used to set the shell’s compatibility level. See Shell Compatibility Mode , for a description of the various compatibility levels and their effects. The value may be a decimal number (e.g., 4.2) or an integer (e.g., 42) corresponding to the desired compatibility level. If BASH_COMPAT is unset or set to the empty string, the compatibility level is set to the default for the current version. If BASH_COMPAT is set to a value that is not one of the valid compatibility levels, the shell prints an error message and sets the compatibility level to the default for the current version. The valid values correspond to the compatibility levels described below (see Shell Compatibility Mode ). For example, 4.2 and 42 are valid values that correspond to the compat42 shopt option and set the compatibility level to 42. The current version is also a valid value.
If this variable is set when Bash is invoked to execute a shell script, its value is expanded and used as the name of a startup file to read before executing the script. See Bash Startup Files .
The command argument to the -c invocation option.
An array variable whose members are the line numbers in source files where each corresponding member of FUNCNAME was invoked. ${BASH_LINENO[$i]} is the line number in the source file ( ${BASH_SOURCE[$i+1]} ) where ${FUNCNAME[$i]} was called (or ${BASH_LINENO[$i-1]} if referenced within another shell function). Use LINENO to obtain the current line number.
A colon-separated list of directories in which the shell looks for dynamically loadable builtins specified by the enable command.
An array variable whose members are assigned by the ‘ =~ ’ binary operator to the [[ conditional command (see Conditional Constructs ). The element with index 0 is the portion of the string matching the entire regular expression. The element with index n is the portion of the string matching the n th parenthesized subexpression.
An array variable whose members are the source filenames where the corresponding shell function names in the FUNCNAME array variable are defined. The shell function ${FUNCNAME[$i]} is defined in the file ${BASH_SOURCE[$i]} and called from ${BASH_SOURCE[$i+1]}
Incremented by one within each subshell or subshell environment when the shell begins executing in that environment. The initial value is 0. If BASH_SUBSHELL is unset, it loses its special properties, even if it is subsequently reset.
A readonly array variable (see Arrays ) whose members hold version information for this instance of Bash. The values assigned to the array members are as follows:
The major version number (the release ).
The minor version number (the version ).
The patch level.
The build version.
The release status (e.g., beta1 ).
The value of MACHTYPE .
The version number of the current instance of Bash.
If set to an integer corresponding to a valid file descriptor, Bash will write the trace output generated when ‘ set -x ’ is enabled to that file descriptor. This allows tracing output to be separated from diagnostic and error messages. The file descriptor is closed when BASH_XTRACEFD is unset or assigned a new value. Unsetting BASH_XTRACEFD or assigning it the empty string causes the trace output to be sent to the standard error. Note that setting BASH_XTRACEFD to 2 (the standard error file descriptor) and then unsetting it will result in the standard error being closed.
Set the number of exited child status values for the shell to remember. Bash will not allow this value to be decreased below a POSIX -mandated minimum, and there is a maximum value (currently 8192) that this may not exceed. The minimum value is system-dependent.
Used by the select command to determine the terminal width when printing selection lists. Automatically set if the checkwinsize option is enabled (see The Shopt Builtin ), or in an interactive shell upon receipt of a SIGWINCH .
An index into ${COMP_WORDS} of the word containing the current cursor position. This variable is available only in shell functions invoked by the programmable completion facilities (see Programmable Completion ).
The current command line. This variable is available only in shell functions and external commands invoked by the programmable completion facilities (see Programmable Completion ).
The index of the current cursor position relative to the beginning of the current command. If the current cursor position is at the end of the current command, the value of this variable is equal to ${#COMP_LINE} . This variable is available only in shell functions and external commands invoked by the programmable completion facilities (see Programmable Completion ).
Set to an integer value corresponding to the type of completion attempted that caused a completion function to be called: TAB , for normal completion, ‘ ? ’, for listing completions after successive tabs, ‘ ! ’, for listing alternatives on partial word completion, ‘ @ ’, to list completions if the word is not unmodified, or ‘ % ’, for menu completion. This variable is available only in shell functions and external commands invoked by the programmable completion facilities (see Programmable Completion ).
The key (or final key of a key sequence) used to invoke the current completion function.
The set of characters that the Readline library treats as word separators when performing word completion. If COMP_WORDBREAKS is unset, it loses its special properties, even if it is subsequently reset.
An array variable consisting of the individual words in the current command line. The line is split into words as Readline would split it, using COMP_WORDBREAKS as described above. This variable is available only in shell functions invoked by the programmable completion facilities (see Programmable Completion ).
An array variable from which Bash reads the possible completions generated by a shell function invoked by the programmable completion facility (see Programmable Completion ). Each array element contains one possible completion.
An array variable created to hold the file descriptors for output from and input to an unnamed coprocess (see Coprocesses ).
An array variable containing the current contents of the directory stack. Directories appear in the stack in the order they are displayed by the dirs builtin. Assigning to members of this array variable may be used to modify directories already in the stack, but the pushd and popd builtins must be used to add and remove directories. Assignment to this variable will not change the current directory. If DIRSTACK is unset, it loses its special properties, even if it is subsequently reset.
If Bash finds this variable in the environment when the shell starts with value ‘ t ’, it assumes that the shell is running in an Emacs shell buffer and disables line editing.
Expanded and executed similarly to BASH_ENV (see Bash Startup Files ) when an interactive shell is invoked in POSIX Mode (see Bash POSIX Mode ).
Each time this parameter is referenced, it expands to the number of seconds since the Unix Epoch as a floating point value with micro-second granularity (see the documentation for the C library function time for the definition of Epoch). Assignments to EPOCHREALTIME are ignored. If EPOCHREALTIME is unset, it loses its special properties, even if it is subsequently reset.
Each time this parameter is referenced, it expands to the number of seconds since the Unix Epoch (see the documentation for the C library function time for the definition of Epoch). Assignments to EPOCHSECONDS are ignored. If EPOCHSECONDS is unset, it loses its special properties, even if it is subsequently reset.
The numeric effective user id of the current user. This variable is readonly.
A colon-separated list of shell patterns (see Pattern Matching ) defining the list of filenames to be ignored by command search using PATH . Files whose full pathnames match one of these patterns are not considered executable files for the purposes of completion and command execution via PATH lookup. This does not affect the behavior of the [ , test , and [[ commands. Full pathnames in the command hash table are not subject to EXECIGNORE . Use this variable to ignore shared library files that have the executable bit set, but are not executable files. The pattern matching honors the setting of the extglob shell option.
The editor used as a default by the -e option to the fc builtin command.
A colon-separated list of suffixes to ignore when performing filename completion. A filename whose suffix matches one of the entries in FIGNORE is excluded from the list of matched filenames. A sample value is ‘ .o:~ ’
An array variable containing the names of all shell functions currently in the execution call stack. The element with index 0 is the name of any currently-executing shell function. The bottom-most element (the one with the highest index) is "main" . This variable exists only when a shell function is executing. Assignments to FUNCNAME have no effect. If FUNCNAME is unset, it loses its special properties, even if it is subsequently reset.
This variable can be used with BASH_LINENO and BASH_SOURCE . Each element of FUNCNAME has corresponding elements in BASH_LINENO and BASH_SOURCE to describe the call stack. For instance, ${FUNCNAME[$i]} was called from the file ${BASH_SOURCE[$i+1]} at line number ${BASH_LINENO[$i]} . The caller builtin displays the current call stack using this information.
If set to a numeric value greater than 0, defines a maximum function nesting level. Function invocations that exceed this nesting level will cause the current command to abort.
A colon-separated list of patterns defining the set of file names to be ignored by filename expansion. If a file name matched by a filename expansion pattern also matches one of the patterns in GLOBIGNORE , it is removed from the list of matches. The pattern matching honors the setting of the extglob shell option.
An array variable containing the list of groups of which the current user is a member. Assignments to GROUPS have no effect. If GROUPS is unset, it loses its special properties, even if it is subsequently reset.
Up to three characters which control history expansion, quick substitution, and tokenization (see History Expansion ). The first character is the history expansion character, that is, the character which signifies the start of a history expansion, normally ‘ ! ’. The second character is the character which signifies ‘quick substitution’ when seen as the first character on a line, normally ‘ ^ ’. The optional third character is the character which indicates that the remainder of the line is a comment when found as the first character of a word, usually ‘ # ’. The history comment character causes history substitution to be skipped for the remaining words on the line. It does not necessarily cause the shell parser to treat the rest of the line as a comment.
The history number, or index in the history list, of the current command. Assignments to HISTCMD are ignored. If HISTCMD is unset, it loses its special properties, even if it is subsequently reset.
A colon-separated list of values controlling how commands are saved on the history list. If the list of values includes ‘ ignorespace ’, lines which begin with a space character are not saved in the history list. A value of ‘ ignoredups ’ causes lines which match the previous history entry to not be saved. A value of ‘ ignoreboth ’ is shorthand for ‘ ignorespace ’ and ‘ ignoredups ’. A value of ‘ erasedups ’ causes all previous lines matching the current line to be removed from the history list before that line is saved. Any value not in the above list is ignored. If HISTCONTROL is unset, or does not include a valid value, all lines read by the shell parser are saved on the history list, subject to the value of HISTIGNORE . The second and subsequent lines of a multi-line compound command are not tested, and are added to the history regardless of the value of HISTCONTROL .
The name of the file to which the command history is saved. The default value is ~/.bash_history .
The maximum number of lines contained in the history file. When this variable is assigned a value, the history file is truncated, if necessary, to contain no more than that number of lines by removing the oldest entries. The history file is also truncated to this size after writing it when a shell exits. If the value is 0, the history file is truncated to zero size. Non-numeric values and numeric values less than zero inhibit truncation. The shell sets the default value to the value of HISTSIZE after reading any startup files.
A colon-separated list of patterns used to decide which command lines should be saved on the history list. Each pattern is anchored at the beginning of the line and must match the complete line (no implicit ‘ * ’ is appended). Each pattern is tested against the line after the checks specified by HISTCONTROL are applied. In addition to the normal shell pattern matching characters, ‘ & ’ matches the previous history line. ‘ & ’ may be escaped using a backslash; the backslash is removed before attempting a match. The second and subsequent lines of a multi-line compound command are not tested, and are added to the history regardless of the value of HISTIGNORE . The pattern matching honors the setting of the extglob shell option.
HISTIGNORE subsumes the function of HISTCONTROL . A pattern of ‘ & ’ is identical to ignoredups , and a pattern of ‘ [ ]* ’ is identical to ignorespace . Combining these two patterns, separating them with a colon, provides the functionality of ignoreboth .
The maximum number of commands to remember on the history list. If the value is 0, commands are not saved in the history list. Numeric values less than zero result in every command being saved on the history list (there is no limit). The shell sets the default value to 500 after reading any startup files.
If this variable is set and not null, its value is used as a format string for strftime to print the time stamp associated with each history entry displayed by the history builtin. If this variable is set, time stamps are written to the history file so they may be preserved across shell sessions. This uses the history comment character to distinguish timestamps from other history lines.
Contains the name of a file in the same format as /etc/hosts that should be read when the shell needs to complete a hostname. The list of possible hostname completions may be changed while the shell is running; the next time hostname completion is attempted after the value is changed, Bash adds the contents of the new file to the existing list. If HOSTFILE is set, but has no value, or does not name a readable file, Bash attempts to read /etc/hosts to obtain the list of possible hostname completions. When HOSTFILE is unset, the hostname list is cleared.
The name of the current host.
A string describing the machine Bash is running on.
Controls the action of the shell on receipt of an EOF character as the sole input. If set, the value denotes the number of consecutive EOF characters that can be read as the first character on an input line before the shell will exit. If the variable exists but does not have a numeric value, or has no value, then the default is 10. If the variable does not exist, then EOF signifies the end of input to the shell. This is only in effect for interactive shells.
The name of the Readline initialization file, overriding the default of ~/.inputrc .
If Bash finds this variable in the environment when the shell starts, it assumes that the shell is running in an Emacs shell buffer and may disable line editing depending on the value of TERM .
Used to determine the locale category for any category not specifically selected with a variable starting with LC_ .
This variable overrides the value of LANG and any other LC_ variable specifying a locale category.
This variable determines the collation order used when sorting the results of filename expansion, and determines the behavior of range expressions, equivalence classes, and collating sequences within filename expansion and pattern matching (see Filename Expansion ).
This variable determines the interpretation of characters and the behavior of character classes within filename expansion and pattern matching (see Filename Expansion ).
This variable determines the locale used to translate double-quoted strings preceded by a ‘ $ ’ (see Locale-Specific Translation ).
This variable determines the locale category used for number formatting.
This variable determines the locale category used for data and time formatting.
The line number in the script or shell function currently executing. If LINENO is unset, it loses its special properties, even if it is subsequently reset.
Used by the select command to determine the column length for printing selection lists. Automatically set if the checkwinsize option is enabled (see The Shopt Builtin ), or in an interactive shell upon receipt of a SIGWINCH .
A string that fully describes the system type on which Bash is executing, in the standard GNU cpu-company-system format.
How often (in seconds) that the shell should check for mail in the files specified in the MAILPATH or MAIL variables. The default is 60 seconds. When it is time to check for mail, the shell does so before displaying the primary prompt. If this variable is unset, or set to a value that is not a number greater than or equal to zero, the shell disables mail checking.
An array variable created to hold the text read by the mapfile builtin when no variable name is supplied.
The previous working directory as set by the cd builtin.
If set to the value 1, Bash displays error messages generated by the getopts builtin command.
A string describing the operating system Bash is running on.
An array variable (see Arrays ) containing a list of exit status values from the processes in the most-recently-executed foreground pipeline (which may contain only a single command).
If this variable is in the environment when Bash starts, the shell enters POSIX mode (see Bash POSIX Mode ) before reading the startup files, as if the --posix invocation option had been supplied. If it is set while the shell is running, Bash enables POSIX mode, as if the command
had been executed. When the shell enters POSIX mode, it sets this variable if it was not already set.
The process ID of the shell’s parent process. This variable is readonly.
If this variable is set, and is an array, the value of each set element is interpreted as a command to execute before printing the primary prompt ( $PS1 ). If this is set but not an array variable, its value is used as a command to execute instead.
If set to a number greater than zero, the value is used as the number of trailing directory components to retain when expanding the \w and \W prompt string escapes (see Controlling the Prompt ). Characters removed are replaced with an ellipsis.
The value of this parameter is expanded like PS1 and displayed by interactive shells after reading a command and before the command is executed.
The value of this variable is used as the prompt for the select command. If this variable is not set, the select command prompts with ‘ #? ’
The value of this parameter is expanded like PS1 and the expanded value is the prompt printed before the command line is echoed when the -x option is set (see The Set Builtin ). The first character of the expanded value is replicated multiple times, as necessary, to indicate multiple levels of indirection. The default is ‘ + ’.
The current working directory as set by the cd builtin.
Each time this parameter is referenced, it expands to a random integer between 0 and 32767. Assigning a value to this variable seeds the random number generator. If RANDOM is unset, it loses its special properties, even if it is subsequently reset.
Any numeric argument given to a Readline command that was defined using ‘ bind -x ’ (see Bash Builtin Commands when it was invoked.
The contents of the Readline line buffer, for use with ‘ bind -x ’ (see Bash Builtin Commands ).
The position of the mark (saved insertion point) in the Readline line buffer, for use with ‘ bind -x ’ (see Bash Builtin Commands ). The characters between the insertion point and the mark are often called the region .
The position of the insertion point in the Readline line buffer, for use with ‘ bind -x ’ (see Bash Builtin Commands ).
The default variable for the read builtin.
This variable expands to the number of seconds since the shell was started. Assignment to this variable resets the count to the value assigned, and the expanded value becomes the value assigned plus the number of seconds since the assignment. The number of seconds at shell invocation and the current time are always determined by querying the system clock. If SECONDS is unset, it loses its special properties, even if it is subsequently reset.
This environment variable expands to the full pathname to the shell. If it is not set when the shell starts, Bash assigns to it the full pathname of the current user’s login shell.
A colon-separated list of enabled shell options. Each word in the list is a valid argument for the -o option to the set builtin command (see The Set Builtin ). The options appearing in SHELLOPTS are those reported as ‘ on ’ by ‘ set -o ’. If this variable is in the environment when Bash starts up, each shell option in the list will be enabled before reading any startup files. This variable is readonly.
Incremented by one each time a new instance of Bash is started. This is intended to be a count of how deeply your Bash shells are nested.
This variable expands to a 32-bit pseudo-random number each time it is referenced. The random number generator is not linear on systems that support /dev/urandom or arc4random , so each returned number has no relationship to the numbers preceding it. The random number generator cannot be seeded, so assignments to this variable have no effect. If SRANDOM is unset, it loses its special properties, even if it is subsequently reset.
The value of this parameter is used as a format string specifying how the timing information for pipelines prefixed with the time reserved word should be displayed. The ‘ % ’ character introduces an escape sequence that is expanded to a time value or other information. The escape sequences and their meanings are as follows; the braces denote optional portions.
A literal ‘ % ’.
The elapsed time in seconds.
The number of CPU seconds spent in user mode.
The number of CPU seconds spent in system mode.
The CPU percentage, computed as (%U + %S) / %R.
The optional p is a digit specifying the precision, the number of fractional digits after a decimal point. A value of 0 causes no decimal point or fraction to be output. At most three places after the decimal point may be specified; values of p greater than 3 are changed to 3. If p is not specified, the value 3 is used.
The optional l specifies a longer format, including minutes, of the form MM m SS . FF s. The value of p determines whether or not the fraction is included.
If this variable is not set, Bash acts as if it had the value
If the value is null, no timing information is displayed. A trailing newline is added when the format string is displayed.
If set to a value greater than zero, TMOUT is treated as the default timeout for the read builtin (see Bash Builtin Commands ). The select command (see Conditional Constructs ) terminates if input does not arrive after TMOUT seconds when input is coming from a terminal.
In an interactive shell, the value is interpreted as the number of seconds to wait for a line of input after issuing the primary prompt. Bash terminates after waiting for that number of seconds if a complete line of input does not arrive.
If set, Bash uses its value as the name of a directory in which Bash creates temporary files for the shell’s use.
The numeric real user id of the current user. This variable is readonly.

How to Assign Variable in Bash
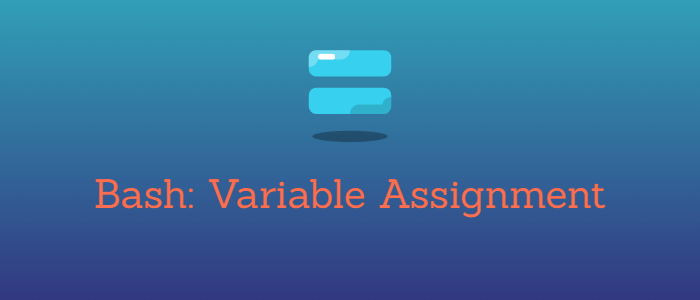
A variable is a named storage location in a program's memory where a value can be stored, retrieved, and manipulated. Like any programming language, Variables are an essential component in the Bash scripts. They allow you to create dynamic scripts that can change based on the data that is passed to them.
In Bash, there are different ways to assign variables which will be covered in detail.
Local vs Global variable assignment
Bash has two types of variables - local, and global based on the scope where they are defined in the script.
Local variable
A local variable is a variable that is defined within a function or a block of code and can only be accessed within that function or block. Local variables are only accessible within the function or block in which they are defined and the value of the variable is lost once the function or the block ends.

Global variable
A global variable, on the other hand, is a variable that is defined outside of any function or block and can be accessed from any part of the program. Global variables are accessible from anywhere in the program, including from within functions and blocks.
See the following script to understand the scope and lifetime of local vs global variables:
In the script:
- num=2 initializes the global num variable.
- local num=1 declared inside the function demo_local_va r block initializes the local num variable whose scope is within the function only
- Outside this function, echoing $num always prints the value of global num variable.

Single variable assignment
In bash, a single variable can be assigned simply using the syntax
with no space before and after the `=` sign. You do not need to declare the data type in bash while assigning a variable.
Integer assignment
To assign an integer to a variable, you can use the following syntax:
In the script, num=1 assigns a global integer variable value 1.

String assignment
There are multiple ways to assign strings:
- Single words can be assigned directly without any quotes.
- Multi-word strings can be defined using Single quotes (') and Double quotes (").
However, there is a difference between how Bash interprets single quotes and double quotes.
When you use single quotes to define a string, it preserves the literal value of each character. On the other hand, if you use double quotes, it recognizes the special symbols ($, `, \) and expands the strings using the shell expansion rules.
In this example,
- greeting2 treats $name as a string and thus it gets expanded to Hello $name .
- While greeting3 expands $name to world , thus generating the string - Hello world .
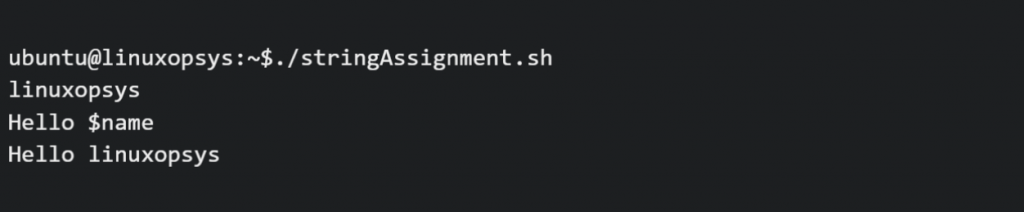
Boolean assignment
To assign a boolean value to a variable, you can use the following syntax:
- true_val=true and false_val=false initializes the boolean variables.
- We can use these booleans in conditional statements to make decisions.

Multi-variable assignments
Bash provides a straightforward syntax for assigning multiple variables in a single line as follows:
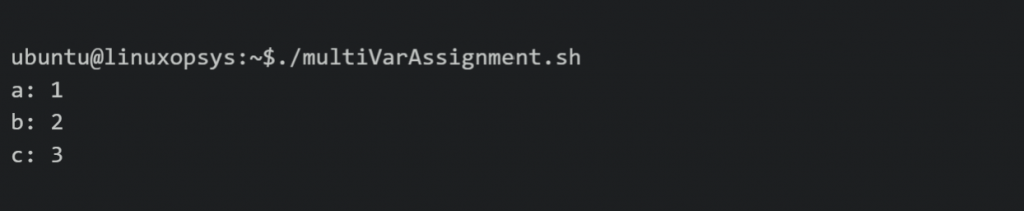
In the above script, a=1, b=2, c=3 initializes three integer variables using a single statement.
Default value assignment
Bash has a built-in syntax for providing default values to the variables:-
If var is not defined, it assigns the defaultval to the newVar.
See the following script to see how default assignments work:
- In the first example, echo $userOutput1 prints 5 to the stdout since var1 is defined.
- In the second example, echo $userOutput2 prints var2 is not defined since var2 is not defined before.
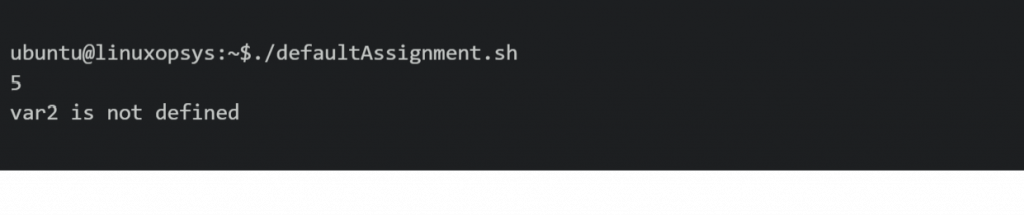
Using let command for assignments
The let command is used to assign numeric values to the variables and perform arithmetic, bitwise, and logical operations.
Consider the following script:
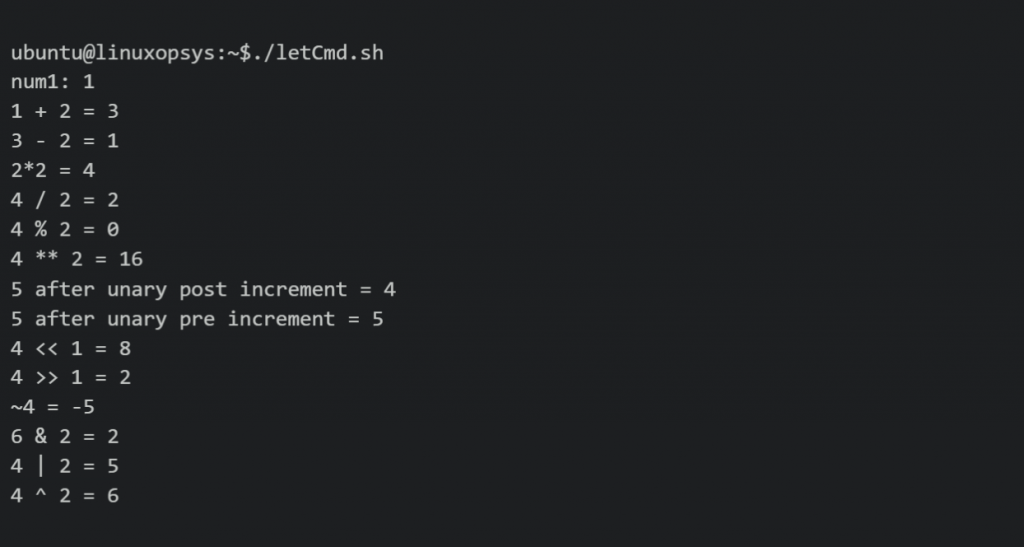
let command is an important utility which provides a simplistic syntax to perform a variety of arithmetic, logical and bitwise operations.
Using Read command
Bash has a built-in read command that can read a line from the standard input (stdin) and split the line into multiple variables.
See the following script to understand how the read command works:

In the script read animal color accepts 2 inputs from the user and stores them in the variables animal and color .
Read command also comes with some useful options:
Prompt flag (-p) helps to provide a useful text to the user and accept inputs. This removes the additional need for echo statements simplifying the code.
Silent flag (-s) helps the user to hide the input from display. This is useful when entering sensitive information such as passwords.
- Input to array
Array (-a) flag reads the input to an array instead of individual words.
- read -p “Enter the username: “ prints the text “Enter the username: “ and also accepts an input from the user.
- read -s -p “Enter the password: “ accepts an input from the user in silent mode, i.e. it is not displayed on the screen.
- read -p “Enter your hobbies(space separated): “ -a hobbies accepts an array input from the user into hobbies variable. The variable can then be iterated over using the array iteration syntax.
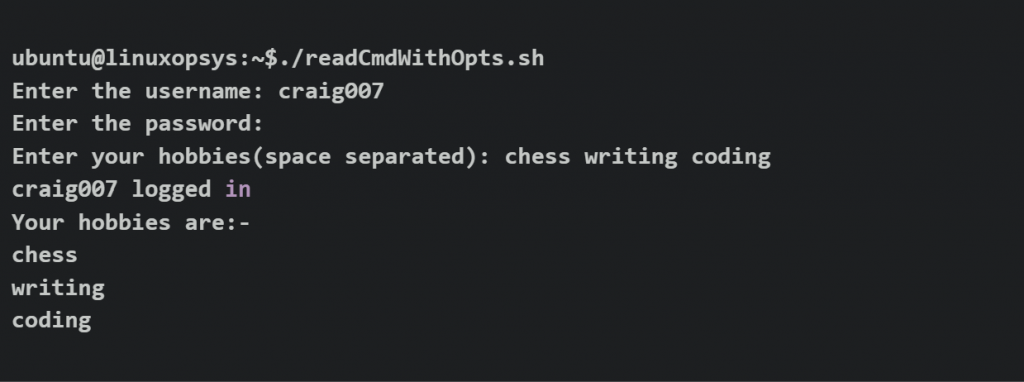
- Bash supports both local and global variables. Using global variables, in general should be avoided since they increase the complexity of the code and may introduce hard to debug bugs.
- Bash provides built-in support for assigning integer, strings and boolean type variables. Data type is not required while assigning variables.
- Multiple variables can be assigned simply by defining multiple variables in a single line.
- Bash also supports initializing variables with default values. This is a good programming practice and helps to avoid bugs due to uninitialized variables.
- The let command is a useful built-in in bash for working with numerics and performing a variety of operations.
- The read command is another important built-in to read input from the command line and create interactive applications.
If this resource helped you, let us know your care by a Thanks Tweet. Tweet a thanks
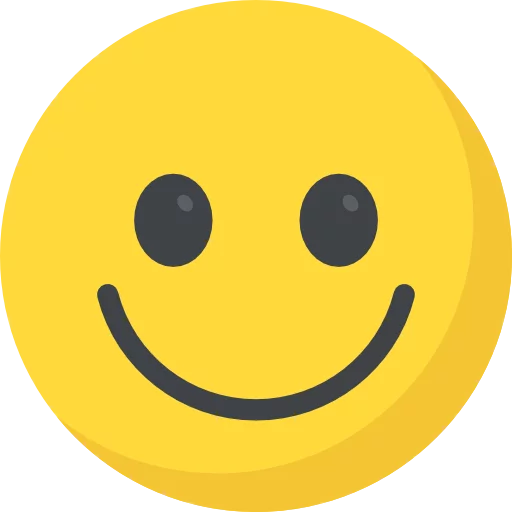
Sorry about that.
Leave a Reply
Leave a comment cancel reply.
- Shell Scripting
- Docker in Linux
- Kubernetes in Linux
- Linux interview question
Shell Scripting – Default Shell Variable Value
A shell gives us an interface to the Unix system. While using an operating system, we indirectly interact with the shell. On Linux distribution systems, each time we use a terminal, we interact with the shell. The job of the shell is to interpret or analyze the Unix commands given by users. A shell accepts commands from the user and transforms them into a form that is understandable to the kernel. In other words, it acts as a mediator between ta user and the kernel unit of the operating system.
This article focuses upon the default shell variable value.
Setting default shell variable value
Undefined variables: A variable that is not defined at the time of declaration is known as an undefined variable.
In this program, we are printing two undefined variables; “myVariable1” and “myVariable2”. In the output, nothing gets printed as these variables are undefined. In simple words, they do not have any initial value.

Undefined variables
We can set variables with default or initial values in shell scripting during echo command with the help of the assignment operator just after the variable name. The default value with which we want to assign the variable, then, comes after the assignment operator. Below is the syntax to set a default value.
Here, myVariable1 and myVariable2 are variable names and value1 and value2 are corresponding default values
If “myVariable1” and “myVariable2” are not initialized then the default values, “value1” and “value2” are substituted respectively. Otherwise, the initialized values of “value1” and “value2” is substituted.
In this program, we have except “myVariable6”, all variables are defined. In simple words, “myVariable6” is initialized whereas other variables are not defined. Thus, myVariable6 has a value initialized at the time of declaration, and other variables have default values.
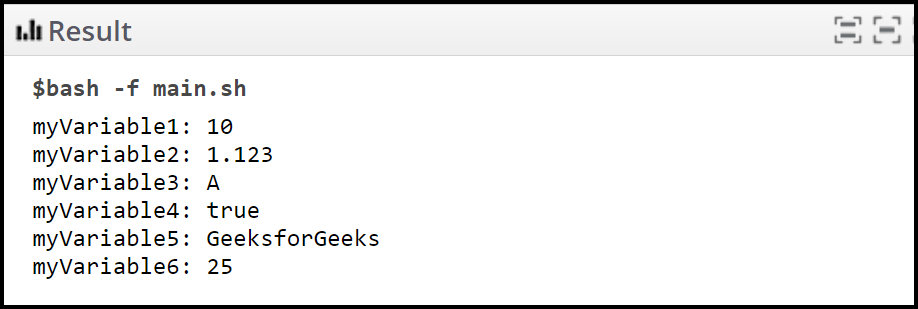
Bash parameter expansion technique is also used to assign variables with default values. In this method also, the variables are assigned with default values during the echo command. But in this case, colon and dash symbols (:-) are used just after the variable name. The default value with which we want to assign the variable, then, comes after these symbols. We can set variables with default or initial values in shell scripting with the help of the below syntax also,
Here, myVariable1 and myVariable2 are variable names, and value1 and value2 are corresponding default values
If “myVariable1” and “myVariable2” are not initialized before then the expansion of “value1” and “value2” is substituted respectively. Otherwise, the initialized value of “value1” and “value2” is substituted.
In this program, we have except “myVariable6”, all variables are defined. In simple words, “myVariable6” is initialized whereas other variables are not defined. Thus, “myVariable6” has a value initialized at the time of declaration, and other variables have default values.
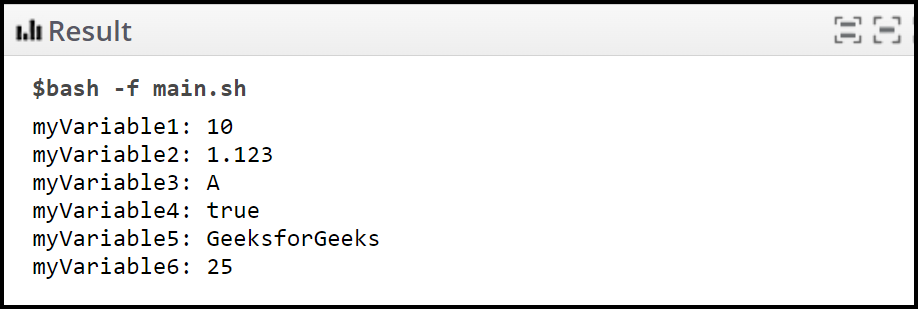
Please Login to comment...
Similar reads.
- Shell Script
- SUMIF in Google Sheets with formula examples
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- bash / FAQ / Shell Scripting
Bash variable assignment examples
by Ramakanta · Published January 19, 2013 · Updated February 11, 2015

This section we will describe the following: 1. Variable assignment 2. Variable substitution 3. Built-in shell variables 4. Other shell variables 5. Variable Assignment
Variable names consist of any number of letters, digits, or underscores. Upper- and lowercase letters are distinct, and names may not start with a digit.
Variables are assigned values using the = operator. There may not be any whitespace between the variable name and the value. You can make multiple assignments on the same line by separating each one with whitespace:
By convention, names for variables used or set by the shell have all uppercase letters; however, you can use uppercase names in your scripts if you use a name that isn’t special to the shell. By default, the shell treats variable values as strings, even if the value of the string is all digits. However, when a value is assigned to an integer variable (created via declare -i), Bash evaluates the righthand side of the assignment as an expression.
For example:
The += operator allows you to add or append the righthand side of the assignment to an existing value. Integer variables treat the righthand side as an expression, which is evaluated and added to the value. Arrays add the new elements to the array.
Variable Substitution
No spaces should be used in the following expressions. The colon (:) is optional; if it’s included, var must be nonnull as well as set.
var=value … Set each variable var to a value.
${var} Use value of var; braces are optional if var is separated from the following text. They are required for array variables.
${var:-value} Use var if set; otherwise, use value.
${var:=value} Use var if set; otherwise, use value and assign value to var.
${var:?value} Use var if set; otherwise, print value and exit (if not interactive). If value isn’t supplied, print the phrase parameter null or not set.
${var:+value} Use value if var is set; otherwise, use nothing.
${#var} Use the length of var.
${#*} Use the number of positional parameters.
${#@} Same as previous.
${var#pattern} Use value of var after removing text matching pattern from the left. Remove the shortest matching piece.
${var##pattern} Same as #pattern, but remove the longest matching piece.
${var%pattern} Use value of var after removing text matching pattern from the right. Remove the shortest matching piece.
${var%%pattern} Same as %pattern, but remove the longest matching piece.
${var^pattern} Convert the case of var to uppercase. The pattern is evaluated as for filename matching. If the first letter of var matches the pattern, it is converted to uppercase. var can be * or @, in which case the positional parameters are modified. var can also be an array subscripted by * or @, in which case the substitution is applied to all the elements of the array.
${var^^pattern} Same as ^pattern, but apply the match to every letter in the string.
${var,pattern} Same as ^pattern, but convert matching characters to lower case. Applies only to the first character in the string.
${var,,pattern} Same as ,pattern, but apply the match to every letter in the string.
${!prefix*},${!prefix@} List of variables whose names begin with prefix.
${var:pos},${var:pos:len} Starting at position pos (0-based) in variable var, extract len characters, or extract rest of string if no len. pos and len may be arithmetic expressions.When var is * or @, the expansion is performed upon the positional parameters. If pos is zero, then $0 is included in the resulting list. Similarly, var can be an array indexed by * or @.
${var/pat/repl} Use value of var, with first match of pat replaced with repl.
${var/pat} Use value of var, with first match of pat deleted.
${var//pat/repl} Use value of var, with every match of pat replaced with repl.
${var/#pat/repl} Use value of var, with match of pat replaced with repl. Match must occur at beginning of the value.
${var/%pat/repl} Use value of var, with match of pat replaced with repl. Match must occur at end of the value.
${!var} Use value of var as name of variable whose value should be used (indirect reference).
Bash provides a special syntax that lets one variable indirectly reference another:
$ greet=”hello, world” Create initial variable
$ friendly_message=greet Aliasing variable
$ echo ${!friendly_message} Use the alias
hello, world Example:
Built-in Shell Variables
Built-in variables are automatically set by the shell and are typically used inside shell scripts. Built-in variables can make use of the variable substitution patterns shown previously. Note that the $ is not actually part of the variable name, although the variable is always referenced this way. The following are
available in any Bourne-compatible shell:
$# Number of command-line arguments.
$- Options currently in effect (supplied on command line or to set). The shell sets some options automatically.
$? Exit value of last executed command.
$$ Process number of the shell.
$! Process number of last background command.
$0 First word; that is, the command name. This will have the full pathname if it was found via a PATH search.
$n Individual arguments on command line (positional parameters).
The Bourne shell allows only nine parameters to be referenced directly (n = 1–9); Bash allows n to be greater than 9 if specified as ${n}.
$*, $@ All arguments on command line ($1 $2 …).
“$*” All arguments on command line as one string (“$1 $2…”). The values are separated by the first character in $IFS.
“$@” All arguments on command line, individually quoted (“$1” “$2” …). Bash automatically sets the following additional variables: $_ Temporary variable; initialized to pathname of script or program being executed. Later, stores the last argument of previous command. Also stores name of matching MAIL file during mail checks.
BASH The full pathname used to invoke this instance of Bash.
BASHOPTS A read-only, colon-separated list of shell options that are currently enabled. Each item in the list is a valid option for shopt -s. If this variable exists in the environment when Bash starts up, it sets the indicated options before executing any startup files.
BASHPID The process ID of the current Bash process. In some cases, this can differ from $$.
BASH_ALIASES Associative array variable. Each element holds an alias defined with the alias command. Adding an element to this array creates a new alias; removing an element removes the corresponding alias.
BASH_ARGC Array variable. Each element holds the number of arguments for the corresponding function or dot-script invocation. Set only in extended debug mode, with shopt –s extdebug. Cannot be unset.
BASH_ARGV An array variable similar to BASH_ARGC. Each element is one of the arguments passed to a function or dot-script. It functions as a stack, with values being pushed on at each call. Thus, the last element is the last argument to the most recent function or script invocation. Set only in extended debug
mode, with shopt -s extdebug. Cannot be unset.
BASH_CMDS Associative array variable. Each element refers to a command in the internal hash table maintained by the hash command. The index is the command name and the value is the full path to the command. Adding an element to this array adds a command to the hash table; removing an element removes the corresponding entry.
BASH_COMMAND The command currently executing or about to be executed. Inside a trap handler, it is the command running when the trap was invoked.
BASH_EXECUTION_STRING The string argument passed to the –c option.
BASH_LINENO Array variable, corresponding to BASH_SOURCE and FUNCNAME. For any given function number i (starting at zero), ${FUNCNAME[i]} was invoked in file ${BASH_SOURCE[i]} on line ${BASH_LINENO[i]}. The information is stored with the most recent function invocation first. Cannot be unset.
BASH_REMATCH Array variable, assigned by the =~ operator of the [[ ]] construct. Index zero is the text that matched the entire pattern. The other
indices are the text matched by parenthesized subexpressions. This variable is read-only.
BASH_SOURCE Array variable, containing source filenames. Each element corresponds to those in FUNCNAME and BASH_LINENO. Cannot be unset.
BASH_SUBSHELL This variable is incremented by one each time a subshell or subshell environment is created.
BASH_VERSINFO[0] The major version number, or release, of Bash.
BASH_VERSINFO[1] The minor version number, or version, of Bash.
BASH_VERSINFO[2] The patch level.
BASH_VERSINFO[3] The build version.
BASH_VERSINFO[4] The release status.
BASH_VERSINFO[5] The machine type; same value as in $MACHTYPE.
BASH_VERSION A string describing the version of Bash.
COMP_CWORD For programmable completion. Index into COMP_WORDS, indicating the current cursor position.
COMP_KEY For programmable completion. The key, or final key in a sequence, that caused the invocation of the current completion function.
COMP_LINE For programmable completion. The current command line.
COMP_POINT For programmable completion. The position of the cursor as a character index in $COMP_LINE.
COMP_TYPE For programmable completion. A character describing the type of programmable completion. The character is one of Tab for normal completion, ? for a completions list after two Tabs, ! for the list of alternatives on partial word completion, @ for completions if the word is modified, or % for menu completion.
COMP_WORDBREAKS For programmable completion. The characters that the readline library treats as word separators when doing word completion.
COMP_WORDS For programmable completion. Array variable containing the individual words on the command line.
COPROC Array variable that holds the file descriptors used for communicating with an unnamed coprocess.
DIRSTACK Array variable, containing the contents of the directory stack as displayed by dirs. Changing existing elements modifies the stack, but only pushd and popd can add or remove elements from the stack.
EUID Read-only variable with the numeric effective UID of the current user.
FUNCNAME Array variable, containing function names. Each element corresponds to those in BASH_SOURCE and BASH_LINENO.
GROUPS Array variable, containing the list of numeric group IDs in which the current user is a member.
HISTCMD The history number of the current command.
HOSTNAME The name of the current host.
HOSTTYPE A string that describes the host system.
LINENO Current line number within the script or function.
MACHTYPE A string that describes the host system in the GNU cpu-company-system format.
MAPFILE Default array for the mapfile and readarray commands.
OLDPWD Previous working directory (set by cd).
OPTARG Value of argument to last option processed by getopts.
OPTIND Numerical index of OPTARG.
OSTYPE A string that describes the operating system.
PIPESTATUS Array variable, containing the exit statuses of the commands in the most recent foreground pipeline.
PPID Process number of this shell’s parent.
PWD Current working directory (set by cd).
RANDOM[=n] Generate a new random number with each reference; start with integer n, if given.
READLINE_LINE For use with bind -x. The contents of the editing buffer are available in this variable.
READLINE_POINT For use with bind -x. The index in $READLINE_LINE of the insertion point.
REPLY Default reply; used by select and read.
SECONDS[=n] Number of seconds since the shell was started, or, if n is given, number of seconds since the assignment + n.
SHELLOPTS A read-only, colon-separated list of shell options (for set -o). If set in the environment at startup, Bash enables each option present in the list before reading any startup files.
SHLVL Incremented by one every time a new Bash starts up.
UID Read-only variable with the numeric real UID of the current user.
Other Shell Variables
The following variables are not automatically set by the shell, although many of them can influence the shell’s behavior. You typically use them in your .bash_profile or .profile file, where you can define them to suit your needs. Variables can be assigned values by issuing commands of the form:
This list includes the type of value expected when defining these variables:
BASH_ENV If set at startup, names a file to be processed for initialization commands. The value undergoes parameter expansion, command substitution, and arithmetic expansion before being interpreted as a filename.
BASH_XTRACEFD=n File descriptor to which Bash writes trace output (from set -x).
CDPATH=dirs Directories searched by cd; allows shortcuts in changing directories; unset by default.
COLUMNS=n Screen’s column width; used in line edit modes and select lists.
COMPREPLY=(words …) Array variable from which Bash reads the possible completions generated by a completion function.
EMACS If the value starts with t, Bash assumes it’s running in an Emacs buffer and disables line editing.
ENV=file Name of script that is executed at startup in POSIX mode or when Bash is invoked as /bin/sh; useful for storing alias and function definitions. For example, ENV=$HOME/.shellrc.
FCEDIT=file Editor used by fc command. The default is /bin/ed when Bash is in POSIX mode. Otherwise, the default is $EDITOR if set, vi if unset.
FIGNORE=patlist Colon-separated list of patterns describing the set of filenames to ignore when doing filename completion.
GLOBIGNORE=patlist Colon-separated list of patterns describing the set of filenames to ignore during pattern matching.
HISTCONTROL=list Colon-separated list of values controlling how commands are saved in the history file. Recognized values are ignoredups, ignorespace, ignoreboth, and erasedups.
HISTFILE=file File in which to store command history.
HISTFILESIZE=n Number of lines to be kept in the history file. This may be different from the number of commands.
HISTIGNORE=list A colon-separated list of patterns that must match the entire command line. Matching lines are not saved in the history file. An unescaped & in a pattern matches the previous history line.
HISTSIZE=n Number of history commands to be kept in the history file.
HISTTIMEFORMAT=string A format string for strftime(3) to use for printing timestamps along with commands from the history command. If set (even if null), Bash saves timestamps in the history file along with the commands.
HOME=dir Home directory; set by login (from /etc/passwd file).
HOSTFILE=file Name of a file in the same format as /etc/hosts that Bash should use to find hostnames for hostname completion.
IFS=’chars’ Input field separators; default is space, Tab, and newline.
IGNOREEOF=n Numeric value indicating how many successive EOF characters must be typed before Bash exits. If null or nonnumeric value, default is 10.
INPUTRC=file Initialization file for the readline library. This overrides the default value of ~/.inputrc.
LANG=locale Default value for locale; used if no LC_* variables are set.
LC_ALL=locale Current locale; overrides LANG and the other LC_* variables.
LC_COLLATE=locale Locale to use for character collation (sorting order).
LC_CTYPE=locale Locale to use for character class functions.
LC_MESSAGES=locale Locale to use for translating $”…” strings.
LC_NUMERIC=locale Locale to use for the decimal-point character.
LC_TIME=locale Locale to use for date and time formats.
LINES=n Screen’s height; used for select lists.
MAIL=file Default file to check for incoming mail; set by login.
MAILCHECK=n Number of seconds between mail checks; default is 600 (10 minutes).
MAILPATH=files One or more files, delimited by a colon, to check for incoming mail. Along with each file, you may supply an optional message that the shell prints when the file increases in size. Messages are separated from the filename by a ? character, and the default message is You have mail in $_. $_ is replaced with the name of the file. For example, you might have MAIL PATH=”$MAIL?Candygram!:/etc/motd?New Login Message” OPTERR=n When set to 1 (the default value), Bash prints error messages from the built-in getopts command.
PATH=dirlist One or more pathnames, delimited by colons, in which to search for commands to execute. The default for many systems is /bin:/usr/bin. On Solaris, the default is /usr/bin:. However, the standard startup scripts change it to /usr/bin:/usr/ucb:/etc:.
POSIXLY_CORRECT=string When set at startup or while running, Bash enters POSIX mode, disabling behavior and modifying features that conflict with the POSIX standard.
PROMPT_COMMAND=command If set, Bash executes this command each time before printing the primary prompt.
PROMPT_DIRTRIM=n Indicates how many trailing directory components to retain for the \w or \W special prompt strings. Elided components are replaced with an ellipsis.
PS1=string Primary prompt string; default is $.
PS2=string Secondary prompt (used in multiline commands); default is >.
PS3=string Prompt string in select loops; default is #?.
PS4=string Prompt string for execution trace (bash –x or set -x); default is +.
SHELL=file Name of user’s default shell (e.g., /bin/sh). Bash sets this if it’s not in the environment at startup.
TERM=string Terminal type.
TIMEFORMAT=string A format string for the output from the time keyword.
TMOUT=n If no command is typed after n seconds, exit the shell. Also affects the read command and the select loop.
TMPDIR=directory Place temporary files created and used by the shell in directory.
auto_resume=list Enables the use of simple strings for resuming stopped jobs. With a value of exact, the string must match a command name exactly. With a value of substring, it can match a substring of the command name.
histchars=chars Two or three characters that control Bash’s csh-style history expansion. The first character signals a history event, the second is the “quick substitution” character, and the third indicates the start of a comment. The default value is !^#.
In case of any ©Copyright or missing credits issue please check CopyRights page for faster resolutions.
Like the article... Share it.
- Click to print (Opens in new window)
- Click to email a link to a friend (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Facebook (Opens in new window)
- Click to share on Tumblr (Opens in new window)
- Click to share on Pocket (Opens in new window)
- Click to share on Telegram (Opens in new window)
CURRENTLY TRENDING...
Tags: bash variable
- Next story Grep Command Tutorial For Unix
- Previous story Bash redirect to file and screen
Leave a Reply Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Sign Up For Our Free Email Newsletter
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
How do I make Bash my default shell on Ubuntu?
I have a .bash_profile in my home directory, but it isn't getting run on login. If I do the following, then things seem to be as I expect:
But normally that all happens on login. Thoughts?
- 3 Also make sure that you don't have a ~/.profile or ~/.bash_login , as only one of the three is sourced. (I forgot the exact order.) – grawity_u1686 Commented Sep 25, 2009 at 15:20
- 4 Why do you have a different question in the title and different one in the body of your post? – pabouk - Ukraine stay strong Commented Nov 9, 2013 at 10:25
9 Answers 9
Enter your password and state the path to the shell you want to use.
For Bash that would be /bin/bash . For Zsh that would be /usr/bin/zsh .
- 19 +1 - not sure why the OP decided that editing the password file was a better choice, but this is the best answer – kdgregory Commented Sep 25, 2009 at 12:51
- 1 Yeah beat me to it, this is the standard way. – user1931 Commented Sep 25, 2009 at 13:49
- 1 And on ubuntu the path to the shell you want to use is... /bin/bash (and /bin/sh is not the same) – Harry Wood Commented Mar 2, 2012 at 1:01
- 70 Or you can use sudo chsh -s /bin/bash username – Oleg Vaskevich Commented Jan 21, 2014 at 4:03
- 26 You must log out and log back in to see this change. – Neil Traft Commented Jul 6, 2014 at 21:59
On top of akira's answer, you can also edit your /etc/passwd file to specify your default shell.
You will find a line like this example:
The shell is specified at the end.
- 8 Better to use the 'chsh' command as suggested by akira -- less chance to screw something up by mistake. – Lars Haugseth Commented Sep 25, 2009 at 13:17
- 5 not to mention 'chsh' is available when you can't write to /etc/passwd – quack quixote Commented Oct 7, 2009 at 11:30
- 1 But if you so have access to modifying the /etc/passwd and you're careful, John's answer is making good use of the tools the system provides. – AJP Commented Apr 26, 2014 at 11:00
- 4 You must log out and log back in to see this change. – Neil Traft Commented Jul 6, 2014 at 22:01
- 2 If you're running a server without user passwords - providing access only through public/private ssh keys ... it also makes a lot of sense. chsh requires a password. – Keith John Hutchison Commented Sep 10, 2015 at 2:42
Enable bash:
Change shell for user:
- 3 (1) What do you mean by “enable bash”? (2) The user wants to change his own login shell on a remote system. Why do you assume that he has sudo access on that system? Why do you provide instructions in terms of changing another user’s login shell? – G-Man Says 'Reinstate Monica' Commented Jan 26, 2018 at 3:24
- 1 Using chsh (as suggested above) did not work for me. This command did! – Per Lindberg Commented Feb 12, 2019 at 9:30
If you somehow don't see your username in the /etc/passwd file [this is the case when your system is under control of some other domain e.g. in IT companies] Or it says "user not found" with chsh option than below process might help you.
The logic behind the below trick -> On Ubuntu, /bin/sh is dash. You can switch your system to using bash. On Ubuntu, /bin/sh is a symbolic link to dash. You can make it a symbolic link to bash instead.To change it, run
sudo dpkg-reconfigure dash
And press No to switch to bash .
Now, go to Terminal->Edit->preferences->Command and tick the checkbox with statement
Run command as login shell
And that's it.
You might check your terminal program. It might be configured to run /bin/sh rather than /bin/bash
Bash executes .bash_profile only for login sessions. .bashrc is executed for all bash sessions, not only login sessions. Try sourcing .bash_profile from .bashrc (avoid circular dependency!) or configuring your terminal program to run /bin/bash -l as a shell program.
- 2 terminal program has nothing to do with the problem because it is the sshd on the remote machine, which spawns the new shell. – akira Commented Sep 26, 2009 at 4:31
To make any shell your default, first verify it is installed and recognized on your computer by looking at the contents of /etc/shells :
Then use chsh to change your shell:
- https://linux.die.net/man/1/cat
- https://linux.die.net/man/1/whoami
- https://linux.die.net/man/5/shells
- https://linux.die.net/man/1/chsh

One alternative is to rename your startup script into .profile. This file is being source by most Unix shells .

There's not enough information in your question for me to say for sure, but I've hit the same problem before. Assuming you've already get /bin/bash set in your password entry, it may be the way your terminal launches.
If you're trying to launch a GUI terminal, say gnome-terminal you may be expecting the shell to read your bash startup files. However, this doesn't happen on Ubuntu and maybe other systems by default.
The way I've fixed it on Ubuntu is to edit the gnome-terminal preferences, and set the startup command to be bash -l . -l is short for --login . This tells bash to startup as as login shell, which causes it to load the startup scripts as you get when logging in via ssh.
I'm sure there's a good rationale for this being the way it is, but I found it surprising and a more than a bit annoying as I share the same profiles across linux, cywgin and macos systems.
I had this same error, and the above answers did not work for me. For some reason my shell was still loading as just /bin/sh instead of /bin/bash and I have the command to in my .profile as it is supposed to be in Ubuntu 16.04 but I also have a blank .bash_profile file that appears to be being read by .bashrc instead of .profile .
So to get everything to work I simply added the command I needed to launch into my .bash_profile file and then everything worked on next ssh.
So I would say if you have all three files: .bashrc , .bash_profile , .profile make sure your .bash_profile file has stuff in it you want to load on login to the shell environment.

You must log in to answer this question.
Not the answer you're looking for browse other questions tagged ubuntu bash ssh ..
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- Rings demanding identity in the categorical context
- How to export a list of Datasets in separate sheets in XLSX?
- Is the spectrum of Hawking radiation identical to that of thermal radiation?
- I am a sailor. I am planning to call my family onboard with me on the ship from the suez
- Whatever happened to Chessmaster?
- Encode a VarInt
- Background for the Elkies-Klagsbrun curve of rank 29
- Why does Jesus give an action of Yahweh as an example of evil?
- Book or novel about an intelligent monolith from space that crashes into a mountain
- Does Vexing Bauble counter taxed 0 mana spells?
- What's the meaning of "running us off an embankment"?
- Monte Carlo Simulation for PSK modulation
- How much of a discount do you get when buying cards on sale?
- How can I automatically save my renders with incremental filenames in Blender?
- Why does a halfing's racial trait lucky specify you must use the next roll?
- What is the meaning of “即采即发”“边采边发”?
- 2 in 1: Twin Puzzle
- Why was this lighting fixture smoking? What do I do about it?
- Cannot open and HTML file stored on RAM-disk with a browser
- Who was the "Dutch author", "Bumstone Bumstone"?
- Is 3 Ohm resistance value of a PCB fuse reasonable?
- Are there any polls on the opinion about Hamas in the broader Arab or Muslim world?
- Image Intelligence concerning alien structures on the moon
- Memory-optimizing arduino code to be able to print all files from SD card
The bash shell and UNIX commands
Chris Paciorek
September 3, 2024
- Newham and Rosenblatt, Learning the bash Shell, 2nd ed.
[This Unit is still under construction as of 2024-08-28.]
1. Shell basics
The shell is the interface between you and the UNIX operating system.
I’ll use ‘UNIX’ to refer to the family of operating systems that descend from the path-breaking UNIX operating system developed at AT&T’s Bell Labs in the 1970s. These include MacOS and various flavors of Linux (e.g., Ubuntu, Debian, CentOS, Fedora).
When you are working in a terminal window (i.e., a window providing the command line interface), you’re interacting with a shell. From the shell you can run UNIX commands such as cp , ls , grep , etc. (as well as start various applications).
Here’s a graphical representation of how the shell relates to various programs, commands, and the operating system.
There are multiple shells ( sh , bash , zsh , csh , tcsh , ksh ). We’ll assume usage of bash , as this is a very commonly-used shell in Linux, plus was the default for Mac OS X until Catalina ( zsh , very similar to bash, is now the default), the SCF machines, and the UC Berkeley campus cluster (Savio). All of the various shells allow you to run UNIX commands.
For your work on this unit, either bash on a Linux machine, the older version of bash on MacOS, or zsh on MacOS (or Linux) are fine. I’ll probably demo everything using bash on a Linux machine, and there are some annoying differences from the older bash on MacOS that may be occasionally confusing (in particular the options to various commands can differ on MacOS).
The Windows PowerShell and old cmd.exe/DOS command interpreter both provide a command-line interface on Windows, but not a UNIX-based one and not interfaces that will be considered here.
UNIX shell commands are designed to each do a specific task really well and really fast. They are modular and composable, so you can build up complicated operations by combining the commands. These tools were designed decades ago, so using the shell might seem old-fashioned, but the shell still lies at the heart of modern scientific computing. By using the shell you can automate your work and make it reproducible. And once you know how to use it, you’ll find that enter commands quickly and without a lot of typing.
2. Using the bash shell
For this Unit, we’ll rely on the bash shell tutorial for the details of how to use the shell. We won’t cover the page on Managing Processes. For the moment, we won’t cover the page on Regular Expressions, but when we talk about string processing and regular expressions in Unit 5, we’ll come back to that material.
3. bash shell examples
Here we’ll work through a few examples to start to give you a feel for using the bash shell to manage your workflows and process data.
First let’s get the files from GitHub to have a set of files we can do interesting things with.
One important note is that most of the shell commands that work with data inside files (in contrast to commands like ls and cd ) work only with text files and not binary files. Also the commands operate on a line-by-line basis.
Our first mission is some basic manipulation of a data file. Suppose we want to get a sense for the number of weather stations in different states using the coop.txt file.
I could have done that in R or Python, but it would have required starting the program up and reading all the data into memory.
If you feel that manually figuring out the position of the state field is inconsistent with our emphasis on programmatic workflows, see the fifth challenge below.
Our second mission : how can I count the number of fields in a CSV file programmatically?
Trouble-shooting: How could the syntax above get the wrong answer?
Extension: We could write a function that can count the number of fields in any file.
Extension: How could I see if all of the lines have the same number of fields?
Our third mission : was the sqlite3 package in the five most recently modified Quarto Markdown files in the units directory?
Notice that man tail indicates it can take input from a FILE or from stdin . Here it uses stdin , so it is gives the last five lines of the output of ls , not the last five lines of the files indicated in that output.
man grep also indicates it can take input from a FILE or from stdin . However, we want grep to operate on the content of the files indicated in stdin. So we use xargs to convert stdin to be recognized as arguments, which then are the FILE inputs to grep .
Here are some of the ways we can pass information from a command to somewhere else:
- Piping allows us to pass information from one command to another command via stdout to stdin.
- also used to create a temporary variable to pass the output from one command as an option or argument (e.g., the FILE argument) of another command
- File redirection operators such as > and >> allow us to pass information from a command into a file.
Our fourth mission : write a function that will move the most recent n files in your Downloads directory to another directory.
In general, we want to start with a specific case, and then generalize to create the function.
Note that the quotes deal with cases where a file has a space in its name.
If we wanted to handle multiple files, we could do it with a loop:
Side note: if we were just moving files from the current working directory and with files without spaces in their names, it should be possible to use tail -n ${1} without the loop.
Our fifth mission : automate the process of determining what Python packages are used in all of the qmd code chunks here and install those packages on a new machine.
You probably wouldn’t want to use this code to accomplish this task in reality - you would want to see if there are packages that can accomplish this. (In the R ecosystem, the renv and packrat packages do this for R projects.) The main point was to illustrate how one can quickly hack together some code to do fairly complicated tasks.
Our sixth mission : suppose I’ve accidentally started a bunch of jobs (perhaps with a for loop in bash!) and need to kill them. (This example uses syntax from the Managing Processes page of the bash tutorial, so it goes beyond what you were asked to read for this Unit.)
4. bash shell challenges
4.1 first challenge.
Consider the file cpds.csv . How would you write a shell command that returns “There are 8 occurrences of the word ‘Belgium’ in this file.”, where ‘8’ should instead be the correct number of times the word occurs.
Extra: make your code into a function that can operate on any file indicated by the user and any word of interest.
4.2 Second challenge
Consider the data in the RTADataSub.csv file. This is a subset of data giving freeway travel times for segments of a freeway in an Australian city. The data are from a kaggle.com competition. We want to try to understand the kinds of data in each field of the file. The following would be particularly useful if the data were in many files or the data were many gigabytes in size.
- First, take the fourth column. Figure out the unique values in that column.
- Next, automate the process of determining if any of the values are non-numeric so that you don’t have to scan through all of the unique values looking for non-numbers. You’ll need to look for the following regular expression pattern [^0-9] , which is interpreted as NOT any of the numbers 0 through 9.
Extra: do it for all the fields, except the first one. Have your code print out the result in a human-readable way understandable by someone who didn’t write the code. For simplicity, you can assume you know the number of fields.
4.3 Third challenge
- For Belgium, determine the minimum unemployment value (field #6) in cpds.csv in a programmatic way.
- Have what is printed out to the screen look like “Belgium 6.2”.
- Now store the unique values of the countries in a variable, first stripping out the quotation marks.
- Figure out how to automate step 1 to do the calculation for all the countries and print to the screen.
- How would you instead store the results in a new file?
4.4 Fourth challenge
Let’s return to the RTADataSub.csv file and the issue of missing values.
- Create a new file without any rows that have an ‘x’ (which indicate a missing value).
- Turn the code into a function that also prints out the number of rows that are being removed and that sends its output to stdout so that it can be used with piping.
- Now modify your function so that the user could provide the missing value string and the input filename.
4.5 Fifth challenge
Consider the coop.txt weather station file.
Figure out how to use grep to tell you the starting position of the state field. Hints: search for a known state-country combination and figure out what flags you can use with grep to print out the “byte offset” for the matched state.
Use that information to automate the first mission where we extracted the state field using cut . You’ll need to do a bit of arithmetic using shell commands.
4.6 Sixth challenge
Here’s an advanced one - you’ll probably need to use sed , but the brief examples of text substitution in the using bash tutorial (or in the demos above) should be sufficient to solve the problem.
Consider a CSV file that has rows that look like this:
While Pandas would be able to handle this using read_csv() , using cut in UNIX won’t work because of the commas embedded within the fields. The challenge is to convert this file to one that we can use cut on, as follows.
Figure out a way to make this into a new delimited file in which the delimiter is not a comma. At least one solution that will work for this particular two-line dataset does not require you to use regular expressions, just simple replacement of fixed patterns.
5. Regular expressions
Regular expressions (“regex”) are a domain-specific language for finding and manipulating patterns of characters and are a key tool used in UNIX commands such as grep , sed , and awk as well as in scripting languages such as Python and R.
For the moment we’ll focus on learning regular expression syntax in the context of the shell, but in Unit 5, we’ll also use regular expressions within Python using the re package.
The basic idea of regular expressions is that they allow us to find matches of strings or patterns in strings, as well as do substitution. Regular expressions are good for tasks such as:
- extracting pieces of text;
- creating variables from information found in text;
- cleaning and transforming text into a uniform format; and
- mining text by treating documents as data.
Please see the bash shell tutorial for a description of regular expressions. I’ll assign a small set of regex problems due as an assignment , and we’ll talk through those problems in class to explore the regex syntax.
Other resources include:
- Here’s a website where you can interactively test regular expressions on example strings .
- Duncan Temple Lang (UC Davis Statistics) has written a nice tutorial covering regular expressions, illustrated in R.
- Sections 9.9 and 11 of Paul Murrell’s book
- The back/second page of RStudio’s stringr cheatsheet has a cheatsheet on regular expressions .
Versions of regular expressions
One thing that can cause headaches is differences in version of regular expression syntax used. As discussed in man grep , extended regular expressions are standard, with basic regular expressions providing less functionality and Perl regular expressions additional functionality.
The bash shell tutorial provides a full documentation of the extended regular expressions syntax, which we’ll focus on here. This syntax should be sufficient for most usage and should be usable in Python and R, but if you notice something funny going on, it might be due to differences between the regular expressions versions.
- In bash, grep -E (or egrep ) enables use of the extended regular expressions.
- In Python, the re package provides syntax “similar to” Perl .
- In R, stringr provides ICU regular expressions (see help(regex) ), which are based on Perl regular expressions.
More details about Perl regular expressions can be found in the regex Wikipedia page .
General principles for working with regex
The syntax is very concise, so it’s helpful to break down individual regular expressions into the component parts to understand them. As Murrell notes, since regex are their own language, it’s a good idea to build up a regex in pieces as a way of avoiding errors just as we would with any computer code. re.findall in Python and str_detect in R’s stringr , as well as regex101.com are particularly useful in seeing what was matched to help in understanding and learning regular expression syntax and debugging your regex. As with many kinds of coding, I find that debugging my regex is usually what takes most of my time.
Challenge problem
Challenge : Let’s think about what regex syntax we would need to detect any number, integer- or real-valued. Let’s start from a test-driven development perspective of writing out test cases including: - various cases we want to detect, - various tricky cases that are not numbers and we don’t want to detect, and - “corner cases” – tricky (perhaps unexpected) cases that might trip us up.
- Find information about your device
- Rename your device
- Change default apps
- System configuration tools
- Configure startup applications
- Configure Windows startup options
- Enable virtualization
Enable virtualization on Windows
Virtualization lets your Windows device emulate a different operating system, like Android or Linux. Enabling virtualization gives you access to a larger library of apps to use and install on your device. If you upgraded your device from Windows 10 to Windows 11, these steps help you enable virtualization.
Note: Many Windows devices already have virtualization enabled, so you might not need to follow these steps.
Before you begin, determine your device model and manufacturer. You'll need this information later in the process.
Step one: Access the UEFI (or BIOS)
Before you begin, we recommend opening this page on a different device. Here's how to get to the UEFI from Windows:
Save your work and close any open apps
Select Start > Settings > System > Recovery > Advanced startup , then select Restart now
Once your PC restarts, you'll see a screen that displays Choose an option. Select Troubleshoot > Advanced options > UEFI Settings > Restart
Your PC will restart again and you'll be in the UEFI utility. At this step, you might see the UEFI referred to as the BIOS on your PC
Step two: Make changes in the UEFI (or BIOS)
The way the UEFI (or BIOS) appears depends on your PC manufacturer. Once you've enabled virtualization and exited the UEFI, your PC will restart.
Important: Only change what you need to in the UEFI (or BIOS). Adjusting other settings might prevent you from accessing Windows.
Instructions based on your PC manufacturer
Choose your PC manufacturer for specific instructions on enabling virtualization. If you don't see your manufacturer listed, refer to your device documentation.
These are external links to the manufacturers' websites.
|
|
---|---|
Acer |
|
Asus | For PCs with AMD processors:
For PCs with Intel processors:
|
Dell |
|
HP |
|
Lenovo |
|
Microsoft | Virtualization is already enabled on Surface devices. |
Instructions based on your UEFI (or BIOS)
If your PC manufacturer isn't listed or you're unsure who it is, you may be able to find instructions for your UEFI (or BIOS). Choose your UEFI firmware developer for specific instructions on enabling virtualization. If you don't see your firmware developer listed, refer to your device documentation.
AMI | Refer to your device's firmware documentation |
Phoenix | Refer to your device's firmware documentation |
Step three: Turn on Virtual Machine Platform in Windows
These instructions might apply if you upgraded your PC from Windows 10 to Windows 11.
Here's how to turn it on:
Select Start , enter Windows features , and select Turn Windows features on or off from the list of results
In the Windows Features window that just opened, find Virtual Machine Platform and select it
Select OK . You might need to restart your PC
For more advanced info or help with troubleshooting, go to Microsoft Docs .

Need more help?
Want more options.
Explore subscription benefits, browse training courses, learn how to secure your device, and more.
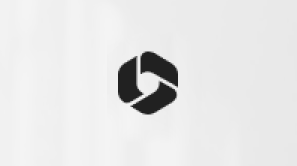
Microsoft 365 subscription benefits
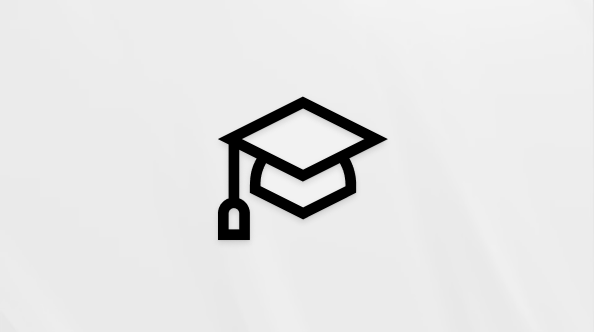
Microsoft 365 training
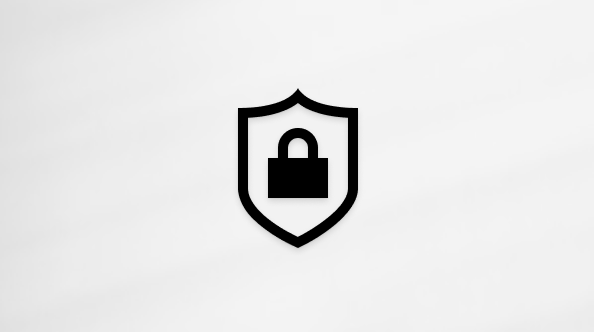
Microsoft security
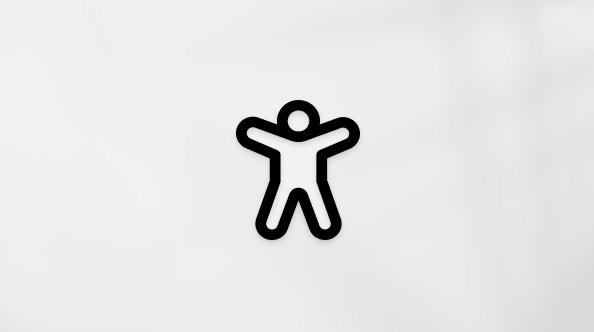
Accessibility center
Communities help you ask and answer questions, give feedback, and hear from experts with rich knowledge.

Ask the Microsoft Community
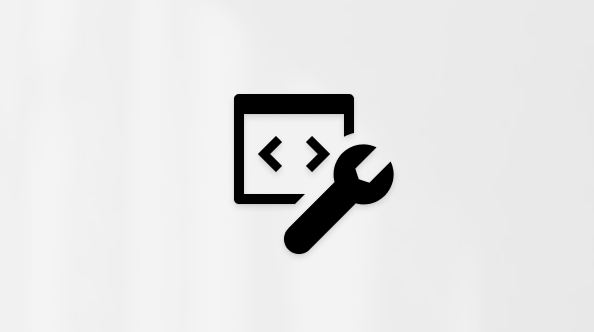
Microsoft Tech Community
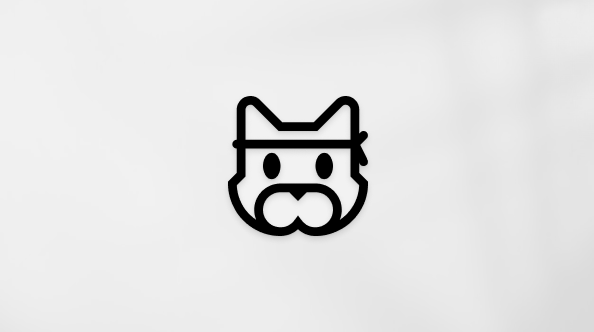
Windows Insiders
Microsoft 365 Insiders
Was this information helpful?
Thank you for your feedback.
5 Linux terminal apps that are better than your default (and why)
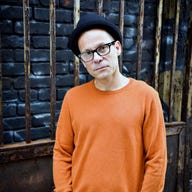
Back in my early days of Linux, the terminal was a necessity. Now, the GUIs are so advanced, user-friendly, and powerful, that you could go your entire Linux career and never touch a terminal window.
Also: 10 Linux keyboard shortcuts I depend on for maximum efficiency
But when you do need to dive into those commands, you'll want a terminal app that's better than the stock default your distribution most likely uses.
Fortunately, there are plenty of options, and here are my five favorites (all of which are free and can be installed from your distribution's default repositories).
Guake is just cool. It's a drop-down terminal client that, when you hit F12 on your keyboard, will roll down from the top of your display. When you're done, hit F12 again and it'll hide itself away until you need it next. Guake is also highly configurable. You can tweak the startup and tabs, the main window, the shell, scrolling, the appearance, keyboard shortcuts, quick open, hooks, and compatibility.
Also: 10 things I always do immediately after installing Linux - and why
Guake includes transparency, theming, shell selection, and much more. What I really like about Guake is that it's always at the ready. Just hit the keyboard shortcut and it's there. Instead of having to add yet another icon to your favorites (or on the desktop), keep it clean while still retaining easy access to your terminal app.
Guake is one of my favorite Linux terminal apps.
Warp is your terminal app on steroids. The big draw to Warp is that it includes a built-in AI that can be used to help you learn commands or even answer questions about the programming language you're trying to learn. One really cool feature found in Warp is that you can ask it questions to figure out the command you need to run.
Also: The first 5 Linux commands every new user should learn
For example, say you want to list out the contents of your home directory with a long list and show hidden files. Instead of having to remember ls -la ~/ , you could type, show what's in ~/ . The natural language addition makes it easy to learn the commands you need to use Linux. I've covered Warp on its own , so you can check out the full review and see just how incredible this app is.
Warp is a brilliant option, especially if you like the idea of AI assistance.
I'm not talking about the PUSA song but, rather, the Linux terminal app. At first blush, you might think Kitty is just a straightforward terminal app but it does have some tricks up its sleeve. For example, Kitty includes split panes which make it possible to split your terminal window into multiple panes (either vertically or horizontally). To split panes hit Ctrl+Shift+Enter on your keyboard and the terminal will split into two vertical panes. Hit the combination again and it'll split the vertical pane with focus into two horizontal panes.
Also: 5 reasons why Linux will overtake Windows and MacOS on the desktop - eventually
Kitty also includes several handy features, such as the ability to edit remote files locally. It's tricky to do this, but once you get the hang of it, it's simple. To pull this off, you have to make use of Kitty's kittens plugins feature, which includes an SSH plugin. Remote into your machine with the help of the ssh kitten like so:
Where user is the username on the remote server and server is the IP address of the remote server. Once logged in, issue the following command:
Now, hit Ctrl+Shift and then click on the file you want to edit. You'll be asked if you want to edit, open, or save the file.
It's tricky, but a really cool feature to make use of.
4. Terminator
Terminator is another terminal app that can do horizontal and vertical splits. You can also assign custom titles to each terminal window, so you never mistake one for another. This is a great feature when using one terminal for admin tasks (say, on a remote machine) and another terminal for local tasks. Those panes can be dragged and dropped into any order.
Terminator also supports profiles, color schemes, font configuration, and customizable keyboard shortcuts.
The panes say, "I'll be back."
Eterm is a hold-over from my Enlightenment days. This terminal window app is fairly basic but it does offer background images, transparency, brightness/contrast/gamma controls, and much more.
The one thing to keep in mind with Eterm is that it was intended for the Enlightenment desktop, so your mileage may vary on what features will actually work, depending on the desktop environment you use.
Also, the menu in the app window will look a bit out of place because it conforms to the Enlightenment look and feel. Even so, this is a fun terminal app to have, even if only for the background image feature.
Eterm holds a special place in my heart.
And there you have it, five terminal apps that you'll most likely enjoy more than the default on your Linux distribution of choice.
These 5 Linux file managers are way better than your default
5 essential linux terms every new user needs to know, the best linux distros for beginners: expert tested.
- Mobile Site
- Staff Directory
- Advertise with Ars
Filter by topic
- Biz & IT
- Gaming & Culture
Front page layout
HELLO, MICROSOFT? YOU THERE? —
“something has gone seriously wrong,” dual-boot systems warn after microsoft update, microsoft said its update wouldn't install on linux devices. it did anyway..
Dan Goodin - Aug 21, 2024 12:17 am UTC
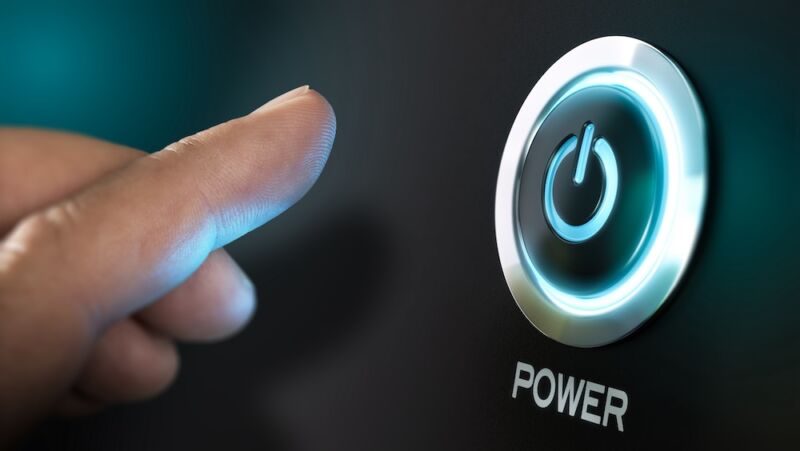
Last Tuesday, loads of Linux users—many running packages released as early as this year—started reporting their devices were failing to boot. Instead, they received a cryptic error message that included the phrase: “Something has gone seriously wrong.”
The cause: an update Microsoft issued as part of its monthly patch release. It was intended to close a 2-year-old vulnerability in GRUB , an open source boot loader used to start up many Linux devices. The vulnerability, with a severity rating of 8.6 out of 10, made it possible for hackers to bypass secure boot, the industry standard for ensuring that devices running Windows or other operating systems don’t load malicious firmware or software during the bootup process. CVE-2022-2601 was discovered in 2022, but for unclear reasons, Microsoft patched it only last Tuesday.
Multiple distros, both new and old, affected
Tuesday’s update left dual-boot devices—meaning those configured to run both Windows and Linux—no longer able to boot into the latter when Secure Boot was enforced. When users tried to load Linux, they received the message: “Verifying shim SBAT data failed: Security Policy Violation. Something has gone seriously wrong: SBAT self-check failed: Security Policy Violation.” Almost immediately support and discussion forums lit up with reports of the failure .
“Note that Windows says this update won't apply to systems that dual-boot Windows and Linux,” one frustrated person wrote. “This obviously isn't true, and likely depends on your system configuration and the distribution being run. It appears to have made some linux efi shim bootloaders incompatible with microcrap efi bootloaders (that's why shifting from MS efi to 'other OS' in efi setup works). It appears that Mint has a shim version that MS SBAT doesn't recognize.”
The reports indicate that multiple distributions, including Debian, Ubuntu, Linux Mint, Zorin OS, and Puppy Linux, are all affected. Microsoft has yet to acknowledge the error publicly, explain how it wasn’t detected during testing, or provide technical guidance to those affected. Company representatives didn’t respond to an email seeking answers.
[ Update: Roughly 17 hours after this post went live, Microsoft supplied the following statement, which also doesn't answer those questions: “This update is not applied when a Linux boot option is detected. We are aware that some secondary boot scenarios are causing issues for some customers, including when using outdated Linux loaders with vulnerable code. We are working with our Linux partners to investigate and address.”]
Microsoft’s bulletin for CVE-20220-2601 explained that the update would install an SBAT —a Linux mechanism for revoking various components in the boot path—but only on devices configured to run only Windows. That way, Secure Boot on Windows devices would no longer be vulnerable to attacks that loaded a GRUB package that exploited the vulnerability. Microsoft assured users their dual-boot systems wouldn’t be affected, although it did warn that devices running older versions of Linux could experience problems.
“The SBAT value is not applied to dual-boot systems that boot both Windows and Linux and should not affect these systems,” the bulletin read. “You might find that older Linux distribution ISOs will not boot. If this occurs, work with your Linux vendor to get an update.”
In fact, the update has been applied to devices that boot both Windows and Linux. That not only includes dual-boot devices but also Windows devices that can boot Linux from an ISO image , a USB drive, or optical media. What’s more, many of the affected systems run recently released Linux versions, including Ubuntu 24.04 and Debian 12.6.0.
With Microsoft maintaining radio silence, those affected by the glitch have been forced to find their own remedies. One option is to access their EFI panel and turn off secure boot. Depending on the security needs of the user, that option may not be acceptable. A better short-term option is to delete the SBAT Microsoft pushed out last Tuesday. This means users will still receive some of the benefits of Secure Boot even if they remain vulnerable to attacks that exploit CVE-2022-2601. The steps for this remedy are outlined here (thanks to manutheeng for the reference).
The specific steps are:
1. Disable Secure Boot 2. Log into your Ubuntu user and open a terminal 3. Delete the SBAT policy with: Code: Select all sudo mokutil --set-sbat-policy delete 4. Reboot your PC and log back into Ubuntu to update the SBAT policy 5. Reboot and then re-enable secure boot in your BIOS.
Further Reading
“At the end of the day, while Secure Boot does make booting Windows more secure, it seems to have a growing pile of flaws that make it not quite as secure as it's intended to be,” said Will Dormann, a senior vulnerability analyst at security firm Analygence. “SecureBoot gets messy in that it's not a MS-only game, though they have the keys to the kingdom. Any vulnerability in a SecureBoot component might affect a SecureBoot-enabled Windows-only system. As such, MS has to address/block vulnerable things.”
reader comments
Promoted comments.

It's unclear to me why Microsoft tried to patch it at all, ever - GRUB is not part of Windows and nothing to do with Microsoft. Why is Windows trying to patch the bootloader of an operating system it knows nothing about? It's not surprising this blew up in everyone's face.
Thank you for a clear(er) explanation - I ran: mokutil --list-sbat-revocations" and got: sbat,1,2021030218 I have no idea what that is from but this laptop (Lenovo, P53) gets updates from Lenovo even on Linux (via LVFS). I will point out also that Microsoft has it's keys baked into the UEFI and so if you put a Microsoft boot disk in this machine, it will happily wipe linux, because it has a valid install key from when the device was manufactured.....
Channel Ars Technica
Aug 2024: A look at the latest Microsoft Entra key feature releases, announcements, and updates

Adam Matthews
August 26th, 2024 0 0
Welcome to the August edition of our monthly developer update, summarizing the latest news and developments in Microsoft Entra. This month, we bring you feature updates, public previews, announcements, and other important updates. These updates aim to bolster your security processes and simplify your workflows.
Read on to learn more and make the most of Microsoft Entra.
What went Generally Available (GA) since Jul 2024?
AD FS Application Migration Wizard: The AD FS application migration wizard simplifies the migration process from AD FS to Microsoft Entra ID. It evaluates AD FS applications for compatibility and guides admins in resolving issues when preparing applications for migration to Microsoft Entra.
Microsoft Entra External ID – easy authentication with Azure App Service: Simplifying the process of configuring authentication and authorization for external-facing apps in Azure App Service. This improvement allows for seamless configuration directly from the App Service authentication setup without switching into the external tenant.
New Public Previews
- Device-based Conditional Access (CA) to Microsoft 365 and Azure resources on Red Hat Enterprise Linux: We are expanding device-based Conditional Access to Red Hat Enterprise Linux. This capability allows users to register their devices with Microsoft Entra ID, enroll into Intune, and satisfy device-based CA policies when accessing their corporate resources.
News, updates, and resources
- Learn what’s new in Microsoft Entra , such as the latest release notes, known issues, bug fixes, deprecation functionality, and upcoming changes. You can find releases specific for Sovereign Clouds on a dedicated release notes page.
Identity blog
ICYMI: An overview of the latest updates in Microsoft Entra for Jul 2024 . Discover how these new capabilities can be integrated into your projects for optimal performance and security.
Integrate Microsoft Entra External ID with your Python Flask applications for secure user authentication. By leveraging External ID, you can securely manage access for external identities like business partners and customers, create custom branded sign-up experiences, and simplify user management. Follow this step-by-step guide to set up and run a sample Flask application using the Microsoft Authentication Library (MSAL).
Unlock the potential of secure and efficient identity management with Microsoft Entra External ID . Focusing on two key scenarios, we show how External ID simplifies the process of managing external identities, including business partners, consumers, and customers. Dive in to discover how you can streamline identity management with straightforward, customizable solutions that cater to diverse end-user segments.
Enhance your application by adding secure user profile editing with Microsoft Entra External ID . Microsoft Entra External ID provides simple integration for secure user authentication and profile management, offering custom branded sign-up experiences and efficient user management. The blog post covers high-level setup steps and links to detailed documentation for further guidance.
Announcing a new learning module that helps you explore Microsoft Entra External ID . This module guides you through building a website for an online grocery store, demonstrating how to use External ID for customer sign-ups and sign-ins. It’s a perfect starting point if you’re new to External ID, offering a hands-on way to create a proof-of-concept project while discovering the platform’s features and capabilities.
PSRule for Azure is a powerful tool designed to validate your Azure Infrastructure as Code (IaC) . PSRule runs checks based on the Well-Architected Framework, helping you ensure your Azure solutions follow security best practices. See how to integrate PSRule into your workflow and catch security issues in your IaC templates early.
- Phishing-resistant authentication in Microsoft Entra ID
Stay connected and informed
This blog post aims to keep you informed and engaged with the latest Microsoft Entra developments, helping you harness these new features and capabilities in your identity development journey.
To learn more or test out features in the Microsoft Entra portfolio, visit our developer center . Make sure you subscribe to the Identity developer blog for more insights and to keep up with the latest on all things Identity. And, follow us on YouTube for video overviews, tutorials, and deep dives.
Stay tuned for more updates and developments in the world of Microsoft Entra!

Adam Matthews | Content Writer
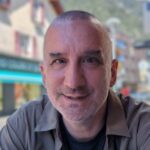
Leave a comment Cancel reply
Log in to start the discussion.

Insert/edit link
Enter the destination URL
Or link to existing content
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
How to write a bash script that takes optional input arguments?
I want my script to be able to take an optional input,
e.g. currently my script is
but I would like it to say:
- parameter-passing

- 3 See also unix.stackexchange.com/questions/122845/… – Vadzim Commented Mar 28, 2016 at 10:12
- 2 ...and stackoverflow.com/questions/2013547/… – Vadzim Commented Mar 28, 2016 at 10:26
- 1 I want to say that the this subject is not about the optional argument but a positional argument with default value. This terminology gives much confusion. "Optional argument" means it would be ok whether those arguments exist in the command line or not. – Joonho Park Commented Feb 16, 2021 at 3:40
10 Answers 10
You could use the default-value syntax:
The above will, as described in Bash Reference Manual - 3.5.3 Shell Parameter Expansion [emphasis mine]:
If parameter is unset or null , the expansion of word is substituted. Otherwise, the value of parameter is substituted.
If you only want to substitute a default value if the parameter is unset (but not if it's null, e.g. not if it's an empty string), use this syntax instead:
Again from Bash Reference Manual - 3.5.3 Shell Parameter Expansion :
Omitting the colon results in a test only for a parameter that is unset. Put another way, if the colon is included, the operator tests for both parameter’s existence and that its value is not null; if the colon is omitted, the operator tests only for existence.
- 81 Please note the semantic difference between the above command, "return foo if $1 is unset or an empty string ", and ${1-foo} , "return foo if $1 is unset". – l0b0 Commented Feb 21, 2012 at 15:12
- 17 Can you explain why this works? Specially, what's the function/purpose of the ':' and '-'? – jwien001 Commented Sep 5, 2014 at 21:11
- 12 @jwein001: In the answer submitted above, a substitution operator is used to return a default value if the variable is undefined. Specifically, the logic is "If $1 exists and isn't null, return its value; otherwise, return foo." The colon is optional. If it's omitted, change "exists and isn't null" to only "exists." The minus sign specifies to return foo without setting $1 equal to 'foo'. Substitution operators are a subclass of expansion operators. See section 6.1.2.1 of Robbins and Beebe's Classic Shell Scripting [O'Reilly] ( shop.oreilly.com/product/9780596005955.do ) – Jubbles Commented Sep 23, 2014 at 20:36
- 6 @Jubbles or if you don't want to buy an entire book for a simple reference... tldp.org/LDP/abs/html/parameter-substitution.html – Ohad Schneider Commented Feb 8, 2017 at 14:39
- 3 This answer would be even better if it showed how to make the default be the result of running a command, as @hagen does (though that answer is inelegant). – sautedman Commented Mar 17, 2017 at 17:47
You can set a default value for a variable like so:
somecommand.sh
Here are some examples of how this works:
- 8 Ah, ok. The - confused me (is it negated?). – khatchad Commented Mar 28, 2018 at 20:58
- 16 Nope - that's just a weird way bash has of doing the assignment. I'll add some more examples to clarify this a bit... thanks! – Brad Parks Commented Mar 29, 2018 at 11:09
- It looks this is the limit of bash argument. Using python terminology, this is not optional, it is positional. It is just a positional argument with default values. Is there no really optional argument in bash? – Joonho Park Commented Feb 17, 2022 at 8:20
- you may be able to use getopts to get what you want - Here's 2 stackoverflow answers that go into greater detail: dfeault values and inside a function – Brad Parks Commented Feb 17, 2022 at 12:47
- Note also, that to confuse even more (with JSON/python dicts) no space is permitted anywhere, e.g. after the colon and/or after/before the dash... where is the principle of least astonishment here?:) – mirekphd Commented May 19, 2022 at 8:39

- 2 Technically, if you pass in an empty string '' that might count as a parameter, but your check will miss it. In that case $# would say how many parameters were given – vmpstr Commented Feb 17, 2012 at 17:40
- 31 -n is the same as ! -z . – l0b0 Commented Feb 21, 2012 at 15:15
- 1 I get different results using -n and ! -z so I would say that is not the case here. – Eliezer Commented Dec 19, 2019 at 21:31
- 6 because you failed to quote the variable, [ -n $1 ] will always be true . If you use bash, [[ -n $1 ]] will behave as you expect, otherwise you must quote [ -n "$1" ] – glenn jackman Commented Mar 19, 2020 at 12:33
- 3 For ones like me: forget the ":" in the code, it's not required, replace it with your real commands! – Anh-Thi DINH Commented Nov 12, 2021 at 13:52
You can check the number of arguments with $#
please don't forget, if its variable $1 .. $n you need write to a regular variable to use the substitution
- 2 Brad's answer above proves that argument variables can also be substituted without intermediate vars. – Vadzim Commented Mar 28, 2016 at 10:05
- 2 +1 for noting the way to use a command like date as the default instead of a fixed value. This is also possible: DAY=${1:-$(date +%F -d "yesterday")} – Garren S Commented Jan 6, 2017 at 21:07
This allows default value for optional 1st arg, and preserves multiple args.

For optional multiple arguments, by analogy with the ls command which can take one or more files or by default lists everything in the current directory:
Does not work correctly for files with spaces in the path, alas. Have not figured out how to make that work yet.
It's possible to use variable substitution to substitute a fixed value or a command (like date ) for an argument. The answers so far have focused on fixed values, but this is what I used to make date an optional argument:
When you use ${1: } you can catch the first parameter (1) passed to your function or(:) you can catch a blank space like a default value.
For example. To be able to use Laravel artisan, I put this into my .bash_aliases file:
and now, I can just type in command line:
- artisan -- and all parameters(${1: } ${2: } ${3: } ${4: } ${5: } ${6: } ${7: }) will be just blank spaces
- artisan cache:clear -- and the first parameter ( ${1: } ) will be cache:clear and all the others will be just blank spaces
So, in this case I can pass 7 parameters optionally.
I hope it can help someone.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged bash arguments parameter-passing or ask your own question .
- The Overflow Blog
- Where does Postgres fit in a world of GenAI and vector databases?
- Mobile Observability: monitoring performance through cracked screens, old...
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Staging Ground Reviewer Motivation
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Can Shatter damage Manifest Mind?
- What is the difference between a "Complaint for Civil Protection Order" and a "Motion for Civil Protection Order"?
- 3D printed teffilin?
- Why does flow separation cause an increase in pressure drag?
- My supervisor wants me to switch to another software/programming language that I am not proficient in. What to do?
- Image Intelligence concerning alien structures on the moon
- What's the meaning of "running us off an embankment"?
- Why did the Fallschirmjäger have such terrible parachutes?
- Why is the movie titled "Sweet Smell of Success"?
- Reusing own code at work without losing licence
- Do the amplitude and frequency of gravitational waves emitted by binary stars change as the stars get closer together?
- What explanations can be offered for the extreme see-sawing in Montana's senate race polling?
- Is it possible to have a planet that's gaslike in some areas and rocky in others?
- Maximizing the common value of both sides of an equation
- How can moral disagreements be resolved when the conflicting parties are guided by fundamentally different value systems?
- Took a pic of old school friend in the 80s who is now quite famous and written a huge selling memoir. Pic has ben used in book without permission
- How can I get the Thevenin equivalent of this circuit?
- What happens if all nine Supreme Justices recuse themselves?
- What is opinion?
- Setting Up Named Credentials For Connecting Two Salesforce Instances i.e. Sandboxes
- How do eradicated diseases make a comeback?
- How would you say a couple of letters (as in mail) if they're not necessarily letters?
- Why does Jesus give an action of Yahweh as an example of evil?
- How to export a list of Datasets in separate sheets in XLSX?

COMMENTS
Copy. We see that both, the x variable and the expression, expand to the default_value string. Likewise, if x is unset, we obtain the same result as with the null string case. Therefore, the default_value string assigns to x, and the expression expands to the new value of x: $ unset x. $ echo "${x:=default_value}"
Very close to what you posted, actually. You can use something called Bash parameter expansion to accomplish this. To get the assigned value, or default if it's missing: FOO="${VARIABLE:-default}" # FOO will be assigned 'default' value if VARIABLE not set or null. # The value of VARIABLE remains untouched.
If the variable is an empty, you can assign a default value. The syntax is: ${var:=defaultValue} Example. Type the following command at a shell prompt: echo ${arg:=Foo} bank= HSBC. echo ${bank:=Citi} unset bank. echo ${bank:=Citi} In this example, the function die assigns a default value if $1 argument is missing:
Method 2: Utilizing the "$ {VARNAME:=default}" Syntax to Assign Default Value. There is another method you can use to assign your default value in the Bash script. In Bash scripting, the " $ {VARNAME:=default} " syntax also allows you to assign a default value to a variable if it is unset or empty.
BASH shell scripting tip: Set default values for variable. If value is not given, the variable is assigned the null string. In shell program it is quite useful to provide default value for variables. For example consider rsync.sh script: $ ./rsync.sh /var/www . It will sync remote /home/vivek directory with local /home/vivek directory.
Now that we've covered these core concepts, let's look at the common techniques for setting default variable values in Bash. 1. Lazy Assignment: ${var:=default} The simplest way to assign a default value is using the := operator: DEST_DIR=${DEST_DIR:=tmp} This will set DEST_DIR to tmp if and only if the variable is currently unset. For example:
Output: Namaste. Bonjour. Bonjour. Using :- will substitute the variable with the default value, whereas := will assign the default value to the variable. In the given example, ${greet:-Namaste} prints out Namaste as ${greet:-Hello} has substituted the greet variable with default value as it was not set. ${greet:=Bonjour} will set the value of ...
Case 05: Default Value Assignment. In Bash, you can assign default values to variables using the ${variable:-default} syntax. Note that this default value assignment does not change the original value of the variable; it only assigns a default value if the variable is empty or unset. Here's a script to learn how it works.
Here, we'll create five variables. The format is to type the name, the equals sign =, and the value. Note there isn't a space before or after the equals sign. Giving a variable a value is often referred to as assigning a value to the variable. We'll create four string variables and one numeric variable, my_name=Dave.
This technique allows for a variable to be assigned a value if another variable is either empty or is undefined. NOTE: This "other variable" can be the same or another variable. excerpt. ${parameter:-word} If parameter is unset or null, the expansion of word is substituted. Otherwise, the value of parameter is substituted.
If the value is null, no timing information is displayed. A trailing newline is added when the format string is displayed. TMOUT ¶. If set to a value greater than zero, TMOUT is treated as the default timeout for the read builtin (see Bash Builtin Commands).The select command (see Conditional Constructs) terminates if input does not arrive after TMOUT seconds when input is coming from a terminal.
Single variable assignment. In bash, a single variable can be assigned simply using the syntax. var_name=<value> with no space before and after the `=` sign. You do not need to declare the data type in bash while assigning a variable. Integer assignment. To assign an integer to a variable, you can use the following syntax:
Method 1: We can set variables with default or initial values in shell scripting during echo command with the help of the assignment operator just after the variable name. The default value with which we want to assign the variable, then, comes after the assignment operator. Below is the syntax to set a default value.
PS4=string Prompt string for execution trace (bash -x or set -x); default is +. SHELL=file Name of user's default shell (e.g., /bin/sh). Bash sets this if it's not in the environment at startup. TERM=string Terminal type. TIMEFORMAT=string A format string for the output from the time keyword.
On Ubuntu, /bin/sh is a symbolic link to dash. You can make it a symbolic link to bash instead.To change it, run. sudo dpkg-reconfigure dash. And press No to switch to bash. Now, go to Terminal->Edit->preferences->Command and tick the checkbox with statement. Run command as login shell. And that's it.
Bash Assign variable from command with default value. Ask Question Asked 9 years, 4 months ago. Modified 9 years, 4 months ago. Viewed 248 times ... Assign default value to variable if one not set, in Bash. 1. default value for a variable in bash script. Hot Network Questions
There are multiple shells (sh, bash, zsh, csh, tcsh, ksh). We'll assume usage of bash, as this is a very commonly-used shell in Linux, plus was the default for Mac OS X until Catalina (zsh, very similar to bash, is now the default), the SCF machines, and the UC Berkeley campus cluster (Savio). All of the various shells allow you to run UNIX ...
Similarly, changing your default browser to the one you use most can speed up your Internet tasks. This article describes the steps to change default programs and app associations in Windows, enhancing your efficiency. How to change the default apps. In the Settings app on your Windows device, select Apps > Default Apps, or use the following ...
Virtualization lets your Windows device emulate a different operating system, like Android or Linux. Enabling virtualization gives you access to a larger library of apps to use and install on your device. If you upgraded your device from Windows 10 to Windows 11, these steps help you enable virtualization.
Fortunately, these Linux commands make it fairly easy to download files from a local or remote location. I'm going to show you three: wget, curl, and scp. 1. wget
5 Linux terminal apps that are better than your default (and why) 5 essential Linux terms every new user needs to know The best Linux distros for beginners: Expert tested
Cerise HUA/Getty Images. Back in my early days of Linux, the terminal was a necessity. Now, the GUIs are so advanced, user-friendly, and powerful, that you could go your entire Linux career and ...
Computer with no Linux gets hacked by attacker who brings grub. Microsoft's patch is to reject grub during the secure boot of a system that does not have Linux and thus finding grub would be ...
90. If you want a way to define defaults in a shell script, use code like this: : ${VAR:="default"} Yes, the line begins with ':'. I use this in shell scripts so I can override variables in ENV, or use the default. This is related because this is my most common use case for that kind of logic. ;]
After you have created a Snippet, you will be able to see it in the Snippets Pane. You can invoke the Snippets Pane by searching for it in the Command Palette and clicking on the "Open snippets pane" option (you can also assign this as a keybinding action in the Settings > Action as well.) To see the command, click on the name of the Snippet.
Rules are evaluated in ascending order of priority. First rule 1 is evaluated, if it's a match the evaluation stops. If no match is found, the next rule is evaluated. If there's no match for any rule, the out of the box Default binary drift rule with default Ignore drift detection applies. Best practices for Drift Detection:
Device-based Conditional Access (CA) to Microsoft 365 and Azure resources on Red Hat Enterprise Linux: We are expanding device-based Conditional Access to Red Hat Enterprise Linux. This capability allows users to register their devices with Microsoft Entra ID, enroll into Intune, and satisfy device-based CA policies when accessing their ...
I was wondering if there is some standard way to have command arguments overwrite variables. For example when defining functions in python it is possible to set a default value. I can see that with an if check I could replace the default with the command line argument, but was unsure if there was a standard way of doing this? -
Role: Depending on your team's requirements, you can change a user's role from Member to Admin or vice versa.For example, you are the team admin and plan to go on leave. You can assign another team member as admin in your absence.; Access: You can assign or change app assignments under the Access column and assign an existing app license or buy and assign a new app license.
1097. You could use the default-value syntax: somecommand ${1:-foo} The above will, as described in Bash Reference Manual - 3.5.3 Shell Parameter Expansion [emphasis mine]: If parameter is unset or null, the expansion of word is substituted. Otherwise, the value of parameter is substituted. If you only want to substitute a default value if the ...